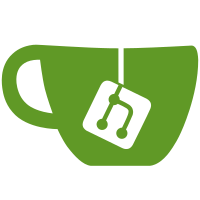
Merge crypto branch. * Makefile.in (pkglib_DATA): Add crypto.lst. (crypto.lst): New target. * commands/hashsum.c: New file. * commands/password.c (check_password): Use grub_crypto_memcmp. * commands/password_pbkdf2.c: New file. * commands/xnu_uuid.c: Remove MD5. Use GRUB_MD_MD5. * conf/any-emu.rmk (grub_emu_SOURCES): Add lib/crypto.c, normal/crypto.c and lib/libgcrypt-grub/cipher/md5.c. (grub_emu_CFLAGS): Add -Wno-missing-field-initializers -Wno-error -I$(srcdir)/lib/libgcrypt_wrap. * conf/common.rmk (normal_mod_SOURCES): Add normal/crypto.c. (pkglib_MODULES): Add crypto.mod, hashsum.mod, pbkdf2.mod and password_pbkdf2.mod. (crypto_mod_SOURCES): New variable. (crypto_mod_CFLAGS): Likewise. (crypto_mod_LDFLAGS): Likewise. (hashsum_mod_SOURCES): New variable. (hashsum_mod_CFLAGS): Likewise. (hashsum_mod_LDFLAGS): Likewise. (pbkdf2_mod_SOURCES): New variable. (pbkdf2_mod_CFLAGS): Likewise. (pbkdf2_mod_LDFLAGS): Likewise. (password_pbkdf2_mod_SOURCES): New variable. (password_pbkdf2_mod_CFLAGS): Likewise. (password_pbkdf2_mod_LDFLAGS): Likewise. (bin_UTILITIES): Add grub-mkpasswd-pbkdf2. (grub_mkpasswd_pbkdf2_SOURCES): New variable. (grub_mkpasswd_pbkdf2_CFLAGS): Likewise. Include conf/gcry.rmk. * include/grub/auth.h: Rewritten. * include/grub/crypto.h: New file. * include/grub/disk.h (grub_disk_dev_id): Add GRUB_DISK_DEVICE_LUKS_ID. * include/grub/normal.h (read_crypto_list): New prototype. * lib/crypto.c: New file. * lib/libgcrypt_wrap/cipher_wrap.h: Likewise. * lib/pbkdf2.c: Likewise. * normal/auth.c (grub_auth_strcmp): Removed. (grub_iswordseparator): Likewise. (grub_auth_strword): Likewise. (is_authenticated): Use grub_strword. (grub_auth_check_authentication): Use grub_strcmp, grub_password_get and grub_strword. Pass entered password to authentication callback. * normal/crypto.c: New file. * normal/main.c: Call read_crypto_list. * util/grub-mkpasswd-pbkdf2.c: New file. * util/import_gcry.py: Generate crypto.lst. Add hash blocklen.
100 lines
3.1 KiB
C
100 lines
3.1 KiB
C
/* xnu_uuid.c - transform 64-bit serial number
|
|
to 128-bit uuid suitable for xnu. */
|
|
/*
|
|
* GRUB -- GRand Unified Bootloader
|
|
* Copyright (C) 1995,1996,1998,1999,2001,2002,
|
|
* 2003, 2009 Free Software Foundation, Inc.
|
|
*
|
|
* GRUB is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* GRUB is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with GRUB. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <grub/types.h>
|
|
#include <grub/misc.h>
|
|
#include <grub/mm.h>
|
|
#include <grub/err.h>
|
|
#include <grub/dl.h>
|
|
#include <grub/device.h>
|
|
#include <grub/disk.h>
|
|
#include <grub/fs.h>
|
|
#include <grub/file.h>
|
|
#include <grub/misc.h>
|
|
#include <grub/env.h>
|
|
#include <grub/command.h>
|
|
#include <grub/i18n.h>
|
|
#include <grub/crypto.h>
|
|
|
|
/* This prefix is used by xnu and boot-132 to hash
|
|
together with volume serial. */
|
|
static grub_uint8_t hash_prefix[16]
|
|
= {0xB3, 0xE2, 0x0F, 0x39, 0xF2, 0x92, 0x11, 0xD6,
|
|
0x97, 0xA4, 0x00, 0x30, 0x65, 0x43, 0xEC, 0xAC};
|
|
|
|
static grub_err_t
|
|
grub_cmd_xnu_uuid (grub_command_t cmd __attribute__ ((unused)),
|
|
int argc, char **args)
|
|
{
|
|
grub_uint64_t serial;
|
|
grub_uint8_t *xnu_uuid;
|
|
char uuid_string[sizeof ("xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")];
|
|
char *ptr;
|
|
grub_uint8_t ctx[GRUB_MD_MD5->contextsize];
|
|
|
|
if (argc < 1)
|
|
return grub_error (GRUB_ERR_BAD_ARGUMENT, "UUID required");
|
|
|
|
serial = grub_cpu_to_be64 (grub_strtoull (args[0], 0, 16));
|
|
|
|
GRUB_MD_MD5->init (&ctx);
|
|
GRUB_MD_MD5->write (&ctx, hash_prefix, sizeof (hash_prefix));
|
|
GRUB_MD_MD5->write (&ctx, &serial, sizeof (serial));
|
|
GRUB_MD_MD5->final (&ctx);
|
|
xnu_uuid = GRUB_MD_MD5->read (&ctx);
|
|
|
|
grub_sprintf (uuid_string,
|
|
"%02x%02x%02x%02x-%02x%02x-%02x%02x-%02x%02x-%02x%02x%02x%02x%02x%02x",
|
|
(unsigned int) xnu_uuid[0], (unsigned int) xnu_uuid[1],
|
|
(unsigned int) xnu_uuid[2], (unsigned int) xnu_uuid[3],
|
|
(unsigned int) xnu_uuid[4], (unsigned int) xnu_uuid[5],
|
|
(unsigned int) ((xnu_uuid[6] & 0xf) | 0x30),
|
|
(unsigned int) xnu_uuid[7],
|
|
(unsigned int) ((xnu_uuid[8] & 0x3f) | 0x80),
|
|
(unsigned int) xnu_uuid[9],
|
|
(unsigned int) xnu_uuid[10], (unsigned int) xnu_uuid[11],
|
|
(unsigned int) xnu_uuid[12], (unsigned int) xnu_uuid[13],
|
|
(unsigned int) xnu_uuid[14], (unsigned int) xnu_uuid[15]);
|
|
for (ptr = uuid_string; *ptr; ptr++)
|
|
*ptr = grub_toupper (*ptr);
|
|
if (argc == 1)
|
|
grub_printf ("%s", uuid_string);
|
|
if (argc > 1)
|
|
grub_env_set (args[1], uuid_string);
|
|
|
|
return GRUB_ERR_NONE;
|
|
}
|
|
|
|
static grub_command_t cmd;
|
|
|
|
|
|
GRUB_MOD_INIT (xnu_uuid)
|
|
{
|
|
cmd = grub_register_command ("xnu_uuid", grub_cmd_xnu_uuid,
|
|
N_("GRUBUUID [VARNAME]"),
|
|
N_("Transform 64-bit UUID to format "
|
|
"suitable for XNU."));
|
|
}
|
|
|
|
GRUB_MOD_FINI (xnu_uuid)
|
|
{
|
|
grub_unregister_command (cmd);
|
|
}
|