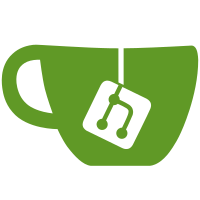
* Add gnulib files generated by gnulib-tool in build-aux, m4 and grub-core/gnulib directories * .bzignore: Add **/.deps and autogenerated gnulib files * configure.ac: Assign auxiliary directory to build-aux, add invocation of gnulib macros, add grub-core/gnulib/Makefile * Makefile.am: Add gnulib directory in SUBDIRS (removing unnecessary .), include m4 directory to aclocal. * Makefile.util.def: Remove direct compilation of gnulib source files and use the new grub-core/gnulib/libgnu.a. * build-aux/config.rpath: move config.rpath from top directory to build-aux * conf/Makefile.common: Remove the macro _GL_UNUSED already defined in gnulib headers * conf/Makefile.extra-dist: Add m4/gnulib-cache.m4 * grub-core/Makefile.core.def: Remove unnecessary extra_dist * grub-core/lib/posix_wrap/localcharset.h (locale_charset): Update header. * grub-core/lib/posix_wrap/langinfo.h (nl_langinfo): Return static string.
71 lines
1.9 KiB
C
71 lines
1.9 KiB
C
/* Formatted output to strings.
|
|
Copyright (C) 2004, 2006-2010 Free Software Foundation, Inc.
|
|
Written by Simon Josefsson and Yoann Vandoorselaere <yoann@prelude-ids.org>.
|
|
|
|
This program is free software; you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation; either version 3, or (at your option)
|
|
any later version.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License along
|
|
with this program; if not, write to the Free Software Foundation,
|
|
Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. */
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include <config.h>
|
|
#endif
|
|
|
|
/* Specification. */
|
|
#include <stdio.h>
|
|
|
|
#include <errno.h>
|
|
#include <limits.h>
|
|
#include <stdarg.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
#include "vasnprintf.h"
|
|
|
|
/* Print formatted output to string STR. Similar to vsprintf, but
|
|
additional length SIZE limit how much is written into STR. Returns
|
|
string length of formatted string (which may be larger than SIZE).
|
|
STR may be NULL, in which case nothing will be written. On error,
|
|
return a negative value. */
|
|
int
|
|
vsnprintf (char *str, size_t size, const char *format, va_list args)
|
|
{
|
|
char *output;
|
|
size_t len;
|
|
size_t lenbuf = size;
|
|
|
|
output = vasnprintf (str, &lenbuf, format, args);
|
|
len = lenbuf;
|
|
|
|
if (!output)
|
|
return -1;
|
|
|
|
if (output != str)
|
|
{
|
|
if (size)
|
|
{
|
|
size_t pruned_len = (len < size ? len : size - 1);
|
|
memcpy (str, output, pruned_len);
|
|
str[pruned_len] = '\0';
|
|
}
|
|
|
|
free (output);
|
|
}
|
|
|
|
if (len > INT_MAX)
|
|
{
|
|
errno = EOVERFLOW;
|
|
return -1;
|
|
}
|
|
|
|
return len;
|
|
}
|