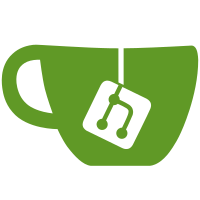
* geninit.sh: New file. * geninitheader.sh: Likewise. * commands/boot.c (grub_boot_init, grub_boot_fini): Removed. * commands/cat.c (grub_cat_init, grub_cat_fini): Likewise. * commands/cmp.c (grub_cmp_init, grub_cmp_fini): Likewise. * commands/configfile.c (grub_configfile_init) (grub_configfile_fini): Likewise. * commands/default.c (grub_default_init, grub_default_fini): Likewise. * commands/help.c (grub_help_init, grub_help_fini): Likewise. * commands/ls.c (grub_ls_init, grub_ls_fini): Likewise. * commands/search.c (grub_search_init, grub_search_fini): Likewise. * commands/terminal.c (grub_terminal_init, grub_terminal_fini): Likewise. * commands/test.c (grub_test_init, grub_test_fini): Likewise. * commands/timeout.c (grub_timeout_init, grub_timeout_fini): Likewise. * commands/i386/pc/halt.c (grub_halt_init, grub_halt_fini): Likewise. * commands/iee1275/halt.c (grub_halt_init, grub_halt_fini): Likewise. * commands/i386/pc/reboot.c (grub_reboot_init, grub_reboot_fini): Likewise. * commands/iee1275/reboot.c (grub_reboot_init, grub_reboot_fini): Likewise. * disk/loopback.c (grub_loop_init, grub_loop_fini): Likewise. * fs/affs.c (grub_affs_init, grub_affs_fini): Likewise. * fs/ext2.c (grub_ext2_init, grub_ext2_fini): Likewise. * fs/fat.c (grub_fat_init, grub_fat_fini): Likewise. * fs/hfs.c (grub_hfs_init, grub_hfs_fini): Likewise. * fs/iso9660.c (grub_iso9660_init, grub_iso9660_fini): Likewise. * fs/jfs.c (grub_jfs_init, grub_jfs_fini): Likewise. * fs/minix.c (grub_minix_init, grub_minix_fini): Likewise. * fs/sfs.c (grub_sfs_init, grub_sfs_fini): Likewise. * fs/ufs.c (grub_ufs_init, grub_ufs_fini): Likewise. * fs/xfs.c (grub_xfs_init, grub_xfs_fini): Likewise. * normal/main.c (grub_normal_init, grub_normal_fini): Likewise. * partmap/amiga.c (grub_amiga_partition_map_init) (grub_amiga_partition_map_fini): Likewise. * partmap/apple.c (grub_apple_partition_map_init) (grub_apple_partition_map_fini): Likewise. * partmap/pc.c (grub_pc_partition_map_init) (grub_pc_partition_map_fini): Likewise. * partmap/sun.c (grub_sun_partition_map_init, grub_sun_partition_map_fini): Likewise. * term/terminfo.c (grub_terminal_init, grub_terminal_fini): Likewise. * util/grub-emu.c: Include <grub_modules_init.h>. (main): Don't initialize and de-initialize any modules directly, use `grub_init_all' and `grub_fini_all' instead. * term/i386/pc/vesafb.c (grub_vesafb_init): Renamed to `grub_vesafb_mod_init'. (grub_vesafb_fini): Renamed to `grub_vesafb_mod_fini'. Updated all users. * term/i386/pc/vga.c (grub_vga_init): Renamed to `grub_vga_mod_init'. Updated all users. (grub_vga_fini): Renamed to `grub_vga_mod_fini'. * conf/i386-pc.rmk (grub_emu_SOURCES): Add `grub_emu_init.c'. (grub_modules_init.lst, grub_modules_init.h, grub_emu_init.c): New rules. * include/grub/dl.h (GRUB_MOD_INIT): Add argument `name'. Generate a function to initialize the module in utilities. Updated all callers. (GRUB_MOD_FINI): Add argument `name'. Generate a function to initialize the module in utilities. Updated all callers.
246 lines
5.1 KiB
C
246 lines
5.1 KiB
C
/*
|
|
* GRUB -- GRand Unified Bootloader
|
|
* Copyright (C) 2003,2005 Free Software Foundation, Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*/
|
|
|
|
#include <grub/file.h>
|
|
#include <grub/misc.h>
|
|
#include <grub/dl.h>
|
|
#include <grub/normal.h>
|
|
#include <grub/types.h>
|
|
#include <grub/mm.h>
|
|
#include <grub/font.h>
|
|
|
|
struct entry
|
|
{
|
|
grub_uint32_t code;
|
|
grub_uint32_t offset;
|
|
};
|
|
|
|
struct font
|
|
{
|
|
struct font *next;
|
|
grub_file_t file;
|
|
grub_uint32_t num;
|
|
struct entry table[0];
|
|
};
|
|
|
|
static struct font *font_list;
|
|
|
|
static int
|
|
add_font (const char *filename)
|
|
{
|
|
grub_file_t file = 0;
|
|
char magic[4];
|
|
grub_uint32_t num, i;
|
|
struct font *font = 0;
|
|
|
|
file = grub_file_open (filename);
|
|
if (! file)
|
|
goto fail;
|
|
|
|
if (grub_file_read (file, magic, 4) != 4)
|
|
goto fail;
|
|
|
|
if (grub_memcmp (magic, GRUB_FONT_MAGIC, 4) != 0)
|
|
{
|
|
grub_error (GRUB_ERR_BAD_FONT, "invalid font magic");
|
|
goto fail;
|
|
}
|
|
|
|
if (grub_file_read (file, (char *) &num, 4) != 4)
|
|
goto fail;
|
|
|
|
num = grub_le_to_cpu32 (num);
|
|
font = (struct font *) grub_malloc (sizeof (struct font)
|
|
+ sizeof (struct entry) * num);
|
|
if (! font)
|
|
goto fail;
|
|
|
|
font->file = file;
|
|
font->num = num;
|
|
|
|
for (i = 0; i < num; i++)
|
|
{
|
|
grub_uint32_t code, offset;
|
|
|
|
if (grub_file_read (file, (char *) &code, 4) != 4)
|
|
goto fail;
|
|
|
|
if (grub_file_read (file, (char *) &offset, 4) != 4)
|
|
goto fail;
|
|
|
|
font->table[i].code = grub_le_to_cpu32 (code);
|
|
font->table[i].offset = grub_le_to_cpu32 (offset);
|
|
}
|
|
|
|
font->next = font_list;
|
|
font_list = font;
|
|
|
|
return 1;
|
|
|
|
fail:
|
|
if (font)
|
|
grub_free (font);
|
|
|
|
if (file)
|
|
grub_file_close (file);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void
|
|
remove_font (struct font *font)
|
|
{
|
|
struct font **p, *q;
|
|
|
|
for (p = &font_list, q = *p; q; p = &(q->next), q = q->next)
|
|
if (q == font)
|
|
{
|
|
*p = q->next;
|
|
|
|
grub_file_close (font->file);
|
|
grub_free (font);
|
|
|
|
break;
|
|
}
|
|
}
|
|
|
|
/* Return the offset of the glyph corresponding to the codepoint CODE
|
|
in the font FONT. If no found, return zero. */
|
|
static grub_uint32_t
|
|
find_glyph (const struct font *font, grub_uint32_t code)
|
|
{
|
|
grub_uint32_t start = 0;
|
|
grub_uint32_t end = font->num - 1;
|
|
struct entry *table = font->table;
|
|
|
|
/* This shouldn't happen. */
|
|
if (font->num == 0)
|
|
return 0;
|
|
|
|
/* Do a binary search. */
|
|
while (start <= end)
|
|
{
|
|
grub_uint32_t i = (start + end) / 2;
|
|
|
|
if (table[i].code < code)
|
|
start = i + 1;
|
|
else if (table[i].code > code)
|
|
end = i - 1;
|
|
else
|
|
return table[i].offset;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
/* Set the glyph to something stupid. */
|
|
static void
|
|
fill_with_default_glyph (unsigned char bitmap[32], unsigned *width)
|
|
{
|
|
if (bitmap)
|
|
{
|
|
unsigned i;
|
|
|
|
for (i = 0; i < 16; i++)
|
|
bitmap[i] = (i & 1) ? 0x55 : 0xaa;
|
|
}
|
|
|
|
*width = 1;
|
|
}
|
|
|
|
/* Get a glyph corresponding to the codepoint CODE. Always fill BITMAP
|
|
and WIDTH with something, even if no glyph is found. */
|
|
int
|
|
grub_font_get_glyph (grub_uint32_t code,
|
|
unsigned char bitmap[32], unsigned *width)
|
|
{
|
|
struct font *font;
|
|
|
|
/* FIXME: It is necessary to cache glyphs! */
|
|
|
|
restart:
|
|
for (font = font_list; font; font = font->next)
|
|
{
|
|
grub_uint32_t offset;
|
|
|
|
offset = find_glyph (font, code);
|
|
if (offset)
|
|
{
|
|
grub_uint32_t w;
|
|
|
|
grub_file_seek (font->file, offset);
|
|
if (grub_file_read (font->file, (char *) &w, 4) != 4)
|
|
{
|
|
remove_font (font);
|
|
goto restart;
|
|
}
|
|
|
|
w = grub_le_to_cpu32 (w);
|
|
if (w != 1 && w != 2)
|
|
{
|
|
/* grub_error (GRUB_ERR_BAD_FONT, "invalid width"); */
|
|
remove_font (font);
|
|
goto restart;
|
|
}
|
|
|
|
if (bitmap
|
|
&& (grub_file_read (font->file, bitmap, w * 16)
|
|
!= (grub_ssize_t) w * 16))
|
|
{
|
|
remove_font (font);
|
|
goto restart;
|
|
}
|
|
|
|
*width = w;
|
|
return 1;
|
|
}
|
|
}
|
|
|
|
/* Uggh... No font was found. */
|
|
fill_with_default_glyph (bitmap, width);
|
|
return 0;
|
|
}
|
|
|
|
static grub_err_t
|
|
font_command (struct grub_arg_list *state __attribute__ ((unused)),
|
|
int argc __attribute__ ((unused)),
|
|
char **args __attribute__ ((unused)))
|
|
{
|
|
if (argc == 0)
|
|
return grub_error (GRUB_ERR_BAD_ARGUMENT, "no font specified");
|
|
|
|
while (argc--)
|
|
if (! add_font (*args++))
|
|
return 1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
GRUB_MOD_INIT(fontmanager)
|
|
{
|
|
(void) mod; /* Stop warning. */
|
|
grub_register_command ("font", font_command, GRUB_COMMAND_FLAG_BOTH,
|
|
"font FILE...",
|
|
"Specify one or more font files to display.", 0);
|
|
}
|
|
|
|
GRUB_MOD_FINI(fontmanager)
|
|
{
|
|
grub_unregister_command ("font");
|
|
}
|