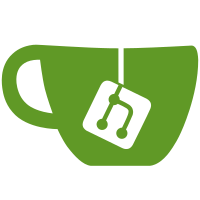
* grub-core/Makefile.core.def (xnu): Add file lzss.c * grub-core/loader/lzss.c: New file. * grub-core/loader/xnu.c (grub_xnu_load_driver): Close binaryfile on Mach-O open failure. * grub-core/loader/macho.c (grub_macho_close): Free uncompressedXX. Don't free cmdsXX in uncompressedXX is set. (grub_macho_file): Init new fields. New argument is_64bit. All users updated. Handle compressed. Error out if no suitable architecture is found. Don't close file. (grub_macho_open): New argument is_64bit. All users updated. * grub-core/loader/macho32.c: Add defines for new fields. * grub-core/loader/macho64.c: Likewise. * grub-core/loader/machoXX.c (grub_macho_contains_macho): Make static. (grub_macho_parse): Handle compressed. Defer actual processing if compressed. (grub_macho_cmds_iterate): Decompress if compressed. New argument "filename". All users updated. (grub_macho_size): New argument "filename". All users updated. (grub_macho_get_entry_point): Likewise. (grub_macho_load): Handle compressed. * include/grub/macho.h (grub_macho_lzss_header): New struct. (GRUB_MACHO_LZSS_OFFSET): New define. (grub_decompress_lzss): New proto. * include/grub/machoload.h (grub_macho_file): New fields to handle compressed. (grub_macho_contains_macho64): Remove proto. (grub_macho_contains_macho32): Likewise. * util/grub.d/30_os-prober.in: Use kernel cache if available.
56 lines
1.8 KiB
C
56 lines
1.8 KiB
C
/**************************************************************
|
|
LZSS.C -- A Data Compression Program
|
|
(tab = 4 spaces)
|
|
***************************************************************
|
|
4/6/1989 Haruhiko Okumura
|
|
Use, distribute, and modify this program freely.
|
|
Please send me your improved versions.
|
|
PC-VAN SCIENCE
|
|
NIFTY-Serve PAF01022
|
|
CompuServe 74050,1022
|
|
**************************************************************/
|
|
|
|
#include <grub/types.h>
|
|
#include <grub/macho.h>
|
|
|
|
#define N 4096 /* size of ring buffer */
|
|
#define F 18 /* upper limit for match_length */
|
|
#define THRESHOLD 2 /* encode string into position and length
|
|
if match_length is greater than this */
|
|
#define NIL N /* index for root of binary search trees */
|
|
|
|
#define EOF -1
|
|
#define getc(file) ((src < srcend) ? *src++ : EOF)
|
|
#define putc(c, file) (dst < dstend) ? (*dst++ = (c)) : 0;
|
|
|
|
grub_size_t
|
|
grub_decompress_lzss (grub_uint8_t *dst, grub_uint8_t *dstend,
|
|
grub_uint8_t *src, grub_uint8_t *srcend)
|
|
{
|
|
int i, j, k, r, c;
|
|
unsigned int flags;
|
|
static unsigned char text_buf[N + F - 1];
|
|
grub_uint8_t *dst0 = dst;
|
|
|
|
for (i = 0; i < N - F; i++) text_buf[i] = ' ';
|
|
r = N - F; flags = 0;
|
|
for ( ; ; ) {
|
|
if (((flags >>= 1) & 256) == 0) {
|
|
if ((c = getc(infile)) == EOF) break;
|
|
flags = c | 0xff00; /* uses higher byte cleverly */
|
|
} /* to count eight */
|
|
if (flags & 1) {
|
|
if ((c = getc(infile)) == EOF) break;
|
|
putc(c, outfile); text_buf[r++] = c; r &= (N - 1);
|
|
} else {
|
|
if ((i = getc(infile)) == EOF) break;
|
|
if ((j = getc(infile)) == EOF) break;
|
|
i |= ((j & 0xf0) << 4); j = (j & 0x0f) + THRESHOLD;
|
|
for (k = 0; k <= j; k++) {
|
|
c = text_buf[(i + k) & (N - 1)];
|
|
putc(c, outfile); text_buf[r++] = c; r &= (N - 1);
|
|
}
|
|
}
|
|
}
|
|
return dst - dst0;
|
|
}
|