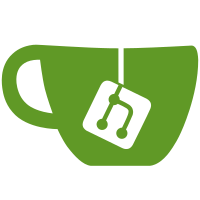
Remove old Stats interface in libcontainers cgroups package. Changed Stats to use unit64 instead of int64 to prevent integer overflow issues. Updated unit tests. Docker-DCO-1.1-Signed-off-by: Vishnu Kannan <vishnuk@google.com> (github: vishh)
66 lines
1.5 KiB
Go
66 lines
1.5 KiB
Go
package fs
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
|
|
"github.com/dotcloud/docker/pkg/libcontainer/cgroups"
|
|
)
|
|
|
|
func TestCpuStats(t *testing.T) {
|
|
helper := NewCgroupTestUtil("cpu", t)
|
|
defer helper.cleanup()
|
|
|
|
const (
|
|
kNrPeriods = 2000
|
|
kNrThrottled = 200
|
|
kThrottledTime = uint64(18446744073709551615)
|
|
)
|
|
|
|
cpuStatContent := fmt.Sprintf("nr_periods %d\n nr_throttled %d\n throttled_time %d\n",
|
|
kNrPeriods, kNrThrottled, kThrottledTime)
|
|
helper.writeFileContents(map[string]string{
|
|
"cpu.stat": cpuStatContent,
|
|
})
|
|
|
|
cpu := &cpuGroup{}
|
|
err := cpu.GetStats(helper.CgroupData, &actualStats)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
expectedStats := cgroups.ThrottlingData{
|
|
Periods: kNrPeriods,
|
|
ThrottledPeriods: kNrThrottled,
|
|
ThrottledTime: kThrottledTime}
|
|
|
|
expectThrottlingDataEquals(t, expectedStats, actualStats.CpuStats.ThrottlingData)
|
|
}
|
|
|
|
func TestNoCpuStatFile(t *testing.T) {
|
|
helper := NewCgroupTestUtil("cpu", t)
|
|
defer helper.cleanup()
|
|
|
|
cpu := &cpuGroup{}
|
|
err := cpu.GetStats(helper.CgroupData, &actualStats)
|
|
if err == nil {
|
|
t.Fatal("Expected to fail, but did not.")
|
|
}
|
|
}
|
|
|
|
func TestInvalidCpuStat(t *testing.T) {
|
|
helper := NewCgroupTestUtil("cpu", t)
|
|
defer helper.cleanup()
|
|
cpuStatContent := `nr_periods 2000
|
|
nr_throttled 200
|
|
throttled_time fortytwo`
|
|
helper.writeFileContents(map[string]string{
|
|
"cpu.stat": cpuStatContent,
|
|
})
|
|
|
|
cpu := &cpuGroup{}
|
|
err := cpu.GetStats(helper.CgroupData, &actualStats)
|
|
if err == nil {
|
|
t.Fatal("Expected failed stat parsing.")
|
|
}
|
|
}
|