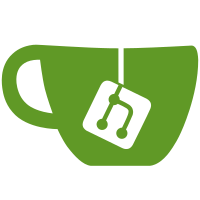
Implement the minimal changes to the local filesystem storage driver and feed them through the distributed storage driver. Create a digest package which contains digest_tools and checksums. Fix the tests to use the new v1 endpoint locations. Fix repository.delete_instance to properly filter the generated queries to avoid most subquery deletes, but still generate them when not explicitly filtered.
48 lines
1.5 KiB
Python
48 lines
1.5 KiB
Python
import unittest
|
|
|
|
from digest.digest_tools import parse_digest, content_path, InvalidDigestException
|
|
|
|
class TestParseDigest(unittest.TestCase):
|
|
def test_parse_good(self):
|
|
examples = [
|
|
('tarsum.v123123+sha1:123deadbeef', (True, 'v123123', 'sha1', '123deadbeef')),
|
|
('tarsum.v1+sha256:123123', (True, 'v1', 'sha256', '123123')),
|
|
('tarsum.v0+md5:abc', (True, 'v0', 'md5', 'abc')),
|
|
('sha1:123deadbeef', (False, None, 'sha1', '123deadbeef')),
|
|
('sha256:123123', (False, None, 'sha256', '123123')),
|
|
('md5:abc', (False, None, 'md5', 'abc')),
|
|
]
|
|
|
|
for digest, output in examples:
|
|
self.assertEquals(parse_digest(digest), output)
|
|
|
|
def test_parse_fail(self):
|
|
examples = [
|
|
'tarsum.v++sha1:123deadbeef',
|
|
'.v1+sha256:123123',
|
|
'tarsum.v+md5:abc',
|
|
'sha1:123deadbeefzxczxv',
|
|
'sha256123123',
|
|
'tarsum.v1+',
|
|
'tarsum.v1123+sha1:',
|
|
]
|
|
|
|
for bad_digest in examples:
|
|
with self.assertRaises(InvalidDigestException):
|
|
parse_digest(bad_digest)
|
|
|
|
|
|
class TestDigestPath(unittest.TestCase):
|
|
def test_paths(self):
|
|
examples = [
|
|
('tarsum.v123123+sha1:123deadbeef', 'tarsum/v123123/sha1/12/123deadbeef'),
|
|
('tarsum.v1+sha256:123123', 'tarsum/v1/sha256/12/123123'),
|
|
('tarsum.v0+md5:abc', 'tarsum/v0/md5/ab/abc'),
|
|
('sha1:123deadbeef', 'sha1/12/123deadbeef'),
|
|
('sha256:123123', 'sha256/12/123123'),
|
|
('md5:abc', 'md5/ab/abc'),
|
|
('md5:1', 'md5/01/1'),
|
|
]
|
|
|
|
for digest, path in examples:
|
|
self.assertEquals(content_path(digest), path)
|