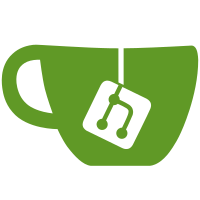
We load stars with the same list API now so that we get the extra metadata needed in the repo list (popularity and last modified)
116 lines
No EOL
3.3 KiB
JavaScript
116 lines
No EOL
3.3 KiB
JavaScript
(function() {
|
|
/**
|
|
* Page that displays details about an user.
|
|
*/
|
|
angular.module('quayPages').config(['pages', function(pages) {
|
|
pages.create('user-view', 'user-view.html', UserViewCtrl, {
|
|
'newLayout': true,
|
|
'title': 'User {{ user.username }}',
|
|
'description': 'User {{ user.username }}'
|
|
})
|
|
}]);
|
|
|
|
function UserViewCtrl($scope, $routeParams, $timeout, ApiService, UserService, UIService, AvatarService) {
|
|
var username = $routeParams.username;
|
|
|
|
$scope.showInvoicesCounter = 0;
|
|
$scope.showAppsCounter = 0;
|
|
$scope.showRobotsCounter = 0;
|
|
$scope.changeEmailInfo = {};
|
|
$scope.changePasswordInfo = {};
|
|
|
|
UserService.updateUserIn($scope);
|
|
|
|
var loadRepositories = function() {
|
|
var options = {
|
|
'sort': true,
|
|
'namespace': username,
|
|
};
|
|
|
|
$scope.repositoriesResource = ApiService.listReposAsResource().withOptions(options).get(function(resp) {
|
|
return resp.repositories;
|
|
});
|
|
};
|
|
|
|
var loadUser = function() {
|
|
$scope.userResource = ApiService.getUserInformationAsResource({'username': username}).get(function(user) {
|
|
$scope.viewuser = user;
|
|
|
|
// Load the repositories.
|
|
$timeout(function() {
|
|
loadRepositories();
|
|
}, 10);
|
|
});
|
|
};
|
|
|
|
// Load the user.
|
|
loadUser();
|
|
|
|
$scope.showRobots = function() {
|
|
$scope.showRobotsCounter++;
|
|
};
|
|
|
|
$scope.showInvoices = function() {
|
|
$scope.showInvoicesCounter++;
|
|
};
|
|
|
|
$scope.showApplications = function() {
|
|
$scope.showAppsCounter++;
|
|
};
|
|
|
|
$scope.changePassword = function() {
|
|
UIService.hidePopover('#changePasswordForm');
|
|
$scope.changePasswordInfo.state = 'changing';
|
|
|
|
var data = {
|
|
'password': $scope.changePasswordInfo.password
|
|
};
|
|
|
|
ApiService.changeUserDetails(data).then(function(resp) {
|
|
$scope.changePasswordInfo.state = 'changed';
|
|
|
|
// Reset the form
|
|
delete $scope.changePasswordInfo['password']
|
|
delete $scope.changePasswordInfo['repeatPassword']
|
|
|
|
// Reload the user.
|
|
UserService.load();
|
|
}, function(result) {
|
|
$scope.changePasswordInfo.state = 'change-error';
|
|
UIService.showFormError('#changePasswordForm', result);
|
|
});
|
|
};
|
|
|
|
$scope.generateClientToken = function() {
|
|
var generateToken = function(password) {
|
|
var data = {
|
|
'password': password
|
|
};
|
|
|
|
ApiService.generateUserClientKey(data).then(function(resp) {
|
|
$scope.generatedClientToken = resp['key'];
|
|
$('#clientTokenModal').modal({});
|
|
}, ApiService.errorDisplay('Could not generate token'));
|
|
};
|
|
|
|
UIService.showPasswordDialog('Enter your password to generate an encrypted version:', generateToken);
|
|
};
|
|
|
|
$scope.changeEmail = function() {
|
|
UIService.hidePopover('#changeEmailForm');
|
|
|
|
var details = {
|
|
'email': $scope.changeEmailInfo.email
|
|
};
|
|
|
|
$scope.changeEmailInfo.state = 'sending';
|
|
ApiService.changeUserDetails(details).then(function() {
|
|
$scope.changeEmailInfo.state = 'sent';
|
|
delete $scope.changeEmailInfo['email'];
|
|
}, function(result) {
|
|
$scope.changeEmailInfo.state = 'send-error';
|
|
UIService.showFormError('#changeEmailForm', result);
|
|
});
|
|
};
|
|
}
|
|
})(); |