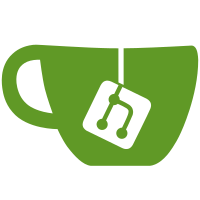
Adds support for creating app repos, viewing app repos and seeing the list of app repos in the Quay UI.
50 lines
No EOL
1.5 KiB
JavaScript
50 lines
No EOL
1.5 KiB
JavaScript
/**
|
|
* An element that displays a list (grid or table) of repositories.
|
|
*/
|
|
angular.module('quay').directive('repoListView', function () {
|
|
var directiveDefinitionObject = {
|
|
priority: 0,
|
|
templateUrl: '/static/directives/repo-list-view.html',
|
|
replace: false,
|
|
transclude: true,
|
|
restrict: 'C',
|
|
scope: {
|
|
namespaces: '=namespaces',
|
|
starredRepositories: '=starredRepositories',
|
|
starToggled: '&starToggled',
|
|
repoKind: '@repoKind'
|
|
},
|
|
controller: function($scope, $element, CookieService) {
|
|
$scope.resources = [];
|
|
$scope.loading = true;
|
|
$scope.showAsList = CookieService.get('quay.repoview') == 'list';
|
|
$scope.optionAllowed = true;
|
|
|
|
$scope.$watch('namespaces', function(namespaces) {
|
|
if (!namespaces) { return; }
|
|
|
|
$scope.loading = false;
|
|
$scope.resources = [];
|
|
namespaces.forEach(function(namespace) {
|
|
if (namespace && namespace.repositories) {
|
|
$scope.resources.push(namespace.repositories);
|
|
if (namespace.repositories.loading) {
|
|
$scope.loading = true;
|
|
}
|
|
}
|
|
});
|
|
|
|
$scope.optionAllowed = $scope.resources.length <= 250;
|
|
if (!$scope.optionAllowed) {
|
|
$scope.showAsList = true;
|
|
}
|
|
}, true);
|
|
|
|
$scope.setShowAsList = function(value) {
|
|
$scope.showAsList = value;
|
|
CookieService.putPermanent('quay.repoview', value ? 'list' : 'grid');
|
|
};
|
|
}
|
|
};
|
|
return directiveDefinitionObject;
|
|
}); |