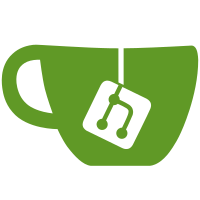
### Description of Changes Issue: https://coreosdev.atlassian.net/browse/QUAY-633 ## Reviewer Checklist - [ ] It works! - [ ] Comments provide sufficient explanations for the next contributor - [ ] Tests cover changes and corner cases - [ ] Follows Quay syntax patterns and format
72 lines
2.5 KiB
Python
72 lines
2.5 KiB
Python
import pytest
|
|
|
|
from mock import patch, ANY, MagicMock
|
|
|
|
from endpoints.api.test.shared import conduct_api_call
|
|
from endpoints.api.repository import RepositoryTrust, Repository
|
|
from endpoints.test.shared import client_with_identity
|
|
from features import FeatureNameValue
|
|
|
|
from test.fixtures import *
|
|
|
|
INVALID_RESPONSE = {
|
|
u'detail': u"u'invalid_req' is not of type 'boolean'",
|
|
u'error_message': u"u'invalid_req' is not of type 'boolean'",
|
|
u'error_type': u'invalid_request',
|
|
u'status': 400,
|
|
u'title': u'invalid_request',
|
|
u'type': u'http://localhost/api/v1/error/invalid_request'
|
|
}
|
|
|
|
NOT_FOUND_RESPONSE = {
|
|
u'detail':
|
|
u'Not Found',
|
|
u'error_message':
|
|
u'Not Found',
|
|
u'error_type':
|
|
u'not_found',
|
|
u'message':
|
|
u'You have requested this URI [/api/v1/repository/devtable/repo/changetrust] but did you mean /api/v1/repository/<apirepopath:repository>/changetrust or /api/v1/repository/<apirepopath:repository>/changevisibility or /api/v1/repository/<apirepopath:repository>/tag/<tag>/images ?',
|
|
u'status':
|
|
404,
|
|
u'title':
|
|
u'not_found',
|
|
u'type':
|
|
u'http://localhost/api/v1/error/not_found'
|
|
}
|
|
|
|
|
|
@pytest.mark.parametrize('trust_enabled,repo_found,expected_body,expected_status', [
|
|
(True, True, {
|
|
'success': True
|
|
}, 200),
|
|
(False, True, {
|
|
'success': True
|
|
}, 200),
|
|
(False, False, NOT_FOUND_RESPONSE, 404),
|
|
('invalid_req', False, INVALID_RESPONSE, 400),
|
|
])
|
|
def test_post_changetrust(trust_enabled, repo_found, expected_body, expected_status, client):
|
|
with patch('endpoints.api.repository.tuf_metadata_api') as mock_tuf:
|
|
with patch(
|
|
'endpoints.api.repository_models_pre_oci.model.repository.get_repository') as mock_model:
|
|
mock_model.return_value = MagicMock() if repo_found else None
|
|
mock_tuf.get_default_tags_with_expiration.return_value = ['tags', 'expiration']
|
|
with client_with_identity('devtable', client) as cl:
|
|
params = {'repository': 'devtable/repo'}
|
|
request_body = {'trust_enabled': trust_enabled}
|
|
assert expected_body == conduct_api_call(cl, RepositoryTrust, 'POST', params, request_body,
|
|
expected_status).json
|
|
|
|
|
|
def test_signing_disabled(client):
|
|
with patch('features.SIGNING', FeatureNameValue('SIGNING', False)):
|
|
with client_with_identity('devtable', client) as cl:
|
|
params = {'repository': 'devtable/simple'}
|
|
response = conduct_api_call(cl, Repository, 'GET', params).json
|
|
assert not response['trust_enabled']
|
|
|
|
|
|
def test_sni_support():
|
|
import ssl
|
|
assert ssl.HAS_SNI
|