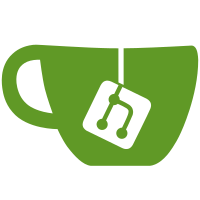
Adds support for creating app repos, viewing app repos and seeing the list of app repos in the Quay UI.
47 lines
No EOL
1.1 KiB
TypeScript
47 lines
No EOL
1.1 KiB
TypeScript
import { Input, Component } from 'angular-ts-decorators';
|
||
|
||
/**
|
||
* A component that displays the icon of a channel.
|
||
*/
|
||
@Component({
|
||
selector: 'channelIcon',
|
||
templateUrl: '/static/js/directives/ui/channel-icon/channel-icon.component.html',
|
||
})
|
||
export class ChannelIconComponent implements ng.IComponentController {
|
||
@Input('<') public name: string;
|
||
|
||
private colors: any;
|
||
|
||
constructor(Config: any, private md5: any) {
|
||
this.colors = Config['CHANNEL_COLORS'];
|
||
}
|
||
|
||
private initial(name: string): string {
|
||
if (name == 'alpha') {
|
||
return 'α';
|
||
}
|
||
if (name == 'beta') {
|
||
return 'β';
|
||
}
|
||
if (name == 'stable') {
|
||
return 'S';
|
||
}
|
||
return name[0].toUpperCase();
|
||
}
|
||
|
||
private color(name: string): string {
|
||
if (name == 'alpha') {
|
||
return this.colors[0];
|
||
}
|
||
if (name == 'beta') {
|
||
return this.colors[1];
|
||
}
|
||
if (name == 'stable') {
|
||
return this.colors[2];
|
||
}
|
||
|
||
var hash: string = this.md5.createHash(name);
|
||
var num: number = parseInt(hash.substr(0, 4));
|
||
return this.colors[num % this.colors.length];
|
||
}
|
||
} |