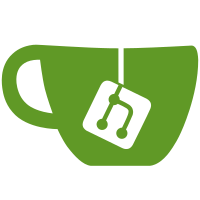
This will ensure that no matter which signature we write for the generated ACI, it is correct for that image.
56 lines
2.1 KiB
Python
56 lines
2.1 KiB
Python
import tarfile
|
|
from util.registry.gzipwrap import GzipWrap
|
|
|
|
class TarImageFormatter(object):
|
|
""" Base class for classes which produce a TAR containing image and layer data. """
|
|
|
|
def build_stream(self, namespace, repository, tag, synthetic_image_id, layer_json,
|
|
get_image_iterator, get_layer_iterator, get_image_json):
|
|
""" Builds and streams a synthetic .tar.gz that represents the formatted TAR created by this
|
|
class's implementation.
|
|
"""
|
|
return GzipWrap(self.stream_generator(namespace, repository, tag,
|
|
synthetic_image_id, layer_json,
|
|
get_image_iterator, get_layer_iterator,
|
|
get_image_json))
|
|
|
|
def stream_generator(self, namespace, repository, tag, synthetic_image_id,
|
|
layer_json, get_image_iterator, get_layer_iterator, get_image_json):
|
|
raise NotImplementedError
|
|
|
|
def tar_file(self, name, contents, mtime=None):
|
|
""" Returns the TAR binary representation for a file with the given name and file contents. """
|
|
length = len(contents)
|
|
tar_data = self.tar_file_header(name, length, mtime=mtime)
|
|
tar_data += contents
|
|
tar_data += self.tar_file_padding(length)
|
|
return tar_data
|
|
|
|
def tar_file_padding(self, length):
|
|
""" Returns TAR file padding for file data of the given length. """
|
|
if length % 512 != 0:
|
|
return '\0' * (512 - (length % 512))
|
|
|
|
return ''
|
|
|
|
def tar_file_header(self, name, file_size, mtime=None):
|
|
""" Returns TAR file header data for a file with the given name and size. """
|
|
info = tarfile.TarInfo(name=name)
|
|
info.type = tarfile.REGTYPE
|
|
info.size = file_size
|
|
|
|
if mtime is not None:
|
|
info.mtime = mtime
|
|
return info.tobuf()
|
|
|
|
def tar_folder(self, name, mtime=None):
|
|
""" Returns TAR file header data for a folder with the given name. """
|
|
info = tarfile.TarInfo(name=name)
|
|
info.type = tarfile.DIRTYPE
|
|
|
|
if mtime is not None:
|
|
info.mtime = mtime
|
|
|
|
# allow the directory to be readable by non-root users
|
|
info.mode = 0755
|
|
return info.tobuf()
|