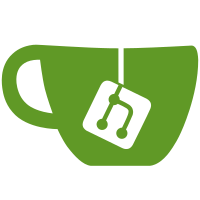
They don't work anyway (since logs will be loaded from random instances), so we just disable them
264 lines
7.8 KiB
JavaScript
264 lines
7.8 KiB
JavaScript
(function() {
|
|
/**
|
|
* The superuser admin page provides a new management UI for Quay Enterprise.
|
|
*/
|
|
angular.module('quayPages').config(['pages', function(pages) {
|
|
pages.create('superuser', 'super-user.html', SuperuserCtrl,
|
|
{
|
|
'newLayout': true,
|
|
'title': 'Quay Enterprise Management'
|
|
})
|
|
}]);
|
|
|
|
function SuperuserCtrl($scope, $location, ApiService, Features, UserService, ContainerService, AngularPollChannel, CoreDialog, TableService) {
|
|
if (!Features.SUPER_USERS) {
|
|
return;
|
|
}
|
|
|
|
// Monitor any user changes and place the current user into the scope.
|
|
UserService.updateUserIn($scope);
|
|
|
|
$scope.multipleInstances = false;
|
|
$scope.configStatus = null;
|
|
$scope.requiresRestart = null;
|
|
$scope.logsCounter = 0;
|
|
$scope.changeLog = null;
|
|
$scope.logsInstance = null;
|
|
$scope.debugServices = null;
|
|
$scope.debugLogs = null;
|
|
$scope.pollChannel = null;
|
|
$scope.logsScrolled = false;
|
|
$scope.csrf_token = encodeURIComponent(window.__token);
|
|
$scope.currentConfig = null;
|
|
$scope.serviceKeysActive = false;
|
|
$scope.globalMessagesActive = false;
|
|
$scope.superUserBuildLogsActive = false;
|
|
$scope.manageUsersActive = false;
|
|
$scope.orderedOrgs = [];
|
|
$scope.orgsPerPage = 10;
|
|
$scope.options = {
|
|
'predicate': 'name',
|
|
'reverse': false,
|
|
'filter': null,
|
|
'page': 0,
|
|
}
|
|
|
|
$scope.loadMessageOfTheDay = function () {
|
|
$scope.globalMessagesActive = true;
|
|
};
|
|
|
|
$scope.loadSuperUserBuildLogs = function () {
|
|
$scope.superUserBuildLogsActive = true;
|
|
};
|
|
|
|
$scope.configurationSaved = function(config) {
|
|
$scope.currentConfig = config;
|
|
$scope.requiresRestart = true;
|
|
};
|
|
|
|
$scope.loadServiceKeys = function() {
|
|
$scope.serviceKeysActive = true;
|
|
};
|
|
|
|
$scope.viewSystemLogs = function(service) {
|
|
if ($scope.pollChannel) {
|
|
$scope.pollChannel.stop();
|
|
}
|
|
|
|
$scope.debugService = service;
|
|
$scope.debugLogs = null;
|
|
|
|
$scope.pollChannel = AngularPollChannel.create($scope, $scope.loadServiceLogs, 2 * 1000 /* 2s */);
|
|
$scope.pollChannel.start();
|
|
};
|
|
|
|
$scope.loadServiceLogs = function(callback) {
|
|
if (!$scope.debugService) { return; }
|
|
|
|
var params = {
|
|
'service': $scope.debugService
|
|
};
|
|
|
|
var errorHandler = ApiService.errorDisplay('Cannot load system logs. Please contact support.',
|
|
function() {
|
|
callback(false);
|
|
});
|
|
|
|
ApiService.getSystemLogs(null, params, /* background */true).then(function(resp) {
|
|
if ($scope.logsInstance != null && $scope.logsInstance != resp['instance']) {
|
|
$scope.multipleInstances = true;
|
|
callback(false);
|
|
return;
|
|
}
|
|
|
|
$scope.logsInstance = resp['instance'];
|
|
$scope.debugLogs = resp['logs'];
|
|
callback(true);
|
|
}, errorHandler);
|
|
};
|
|
|
|
$scope.loadDebugServices = function() {
|
|
if ($scope.pollChannel) {
|
|
$scope.pollChannel.stop();
|
|
}
|
|
|
|
$scope.debugService = null;
|
|
|
|
ApiService.listSystemLogServices().then(function(resp) {
|
|
if ($scope.logsInstance != null && $scope.logsInstance != resp['instance']) {
|
|
$scope.multipleInstances = true;
|
|
callback(false);
|
|
return;
|
|
}
|
|
|
|
$scope.logsInstance = resp['instance'];
|
|
$scope.debugServices = resp['services'];
|
|
}, ApiService.errorDisplay('Cannot load system logs. Please contact support.'))
|
|
};
|
|
|
|
$scope.getChangeLog = function() {
|
|
if ($scope.changeLog) { return; }
|
|
|
|
ApiService.getChangeLog().then(function(resp) {
|
|
$scope.changeLog = resp;
|
|
}, ApiService.errorDisplay('Cannot load change log. Please contact support.'))
|
|
};
|
|
|
|
$scope.loadUsageLogs = function() {
|
|
$scope.logsCounter++;
|
|
};
|
|
|
|
$scope.loadOrganizations = function() {
|
|
if ($scope.organizations) {
|
|
return;
|
|
}
|
|
|
|
$scope.loadOrganizationsInternal();
|
|
};
|
|
|
|
var sortOrgs = function() {
|
|
if (!$scope.organizations) {return;}
|
|
$scope.orderedOrgs = TableService.buildOrderedItems($scope.organizations, $scope.options,
|
|
['name', 'email'], []);
|
|
};
|
|
|
|
$scope.loadOrganizationsInternal = function() {
|
|
$scope.organizationsResource = ApiService.listAllOrganizationsAsResource().get(function(resp) {
|
|
$scope.organizations = resp['organizations'];
|
|
sortOrgs();
|
|
return $scope.organizations;
|
|
});
|
|
};
|
|
|
|
$scope.loadUsers = function() {
|
|
$scope.manageUsersActive = true;
|
|
};
|
|
|
|
$scope.tablePredicateClass = function(name, predicate, reverse) {
|
|
if (name != predicate) {
|
|
return '';
|
|
}
|
|
return 'current ' + (reverse ? 'reversed' : '');
|
|
};
|
|
|
|
$scope.orderBy = function(predicate) {
|
|
if (predicate == $scope.options.predicate) {
|
|
$scope.options.reverse = !$scope.options.reverse;
|
|
return;
|
|
}
|
|
$scope.options.reverse = false;
|
|
$scope.options.predicate = predicate;
|
|
};
|
|
$scope.askDeleteOrganization = function(org) {
|
|
bootbox.confirm('Are you sure you want to delete this organization? Its data will be deleted with it.',
|
|
function(result) {
|
|
if (!result) { return; }
|
|
|
|
var params = {
|
|
'name': org.name
|
|
};
|
|
|
|
ApiService.deleteOrganization(null, params).then(function(resp) {
|
|
$scope.loadOrganizationsInternal();
|
|
}, ApiService.errorDisplay('Could not delete organization'));
|
|
});
|
|
};
|
|
|
|
$scope.askRenameOrganization = function(org) {
|
|
bootbox.prompt('Enter a new name for the organization:', function(newName) {
|
|
if (!newName) { return; }
|
|
|
|
var params = {
|
|
'name': org.name
|
|
};
|
|
|
|
var data = {
|
|
'name': newName
|
|
};
|
|
|
|
ApiService.changeOrganization(data, params).then(function(resp) {
|
|
$scope.loadOrganizationsInternal();
|
|
org.name = newName;
|
|
}, ApiService.errorDisplay('Could not rename organization'));
|
|
});
|
|
};
|
|
|
|
$scope.restartContainer = function() {
|
|
$('#restartingContainerModal').modal({
|
|
keyboard: false,
|
|
backdrop: 'static'
|
|
});
|
|
|
|
ContainerService.restartContainer(function() {
|
|
$scope.checkStatus()
|
|
});
|
|
};
|
|
|
|
$scope.askTakeOwnership = function (entity) {
|
|
$scope.takeOwnershipInfo = {
|
|
'entity': entity
|
|
};
|
|
};
|
|
|
|
$scope.takeOwnership = function (info, callback) {
|
|
var errorDisplay = ApiService.errorDisplay('Could not take ownership of namespace', callback);
|
|
var params = {
|
|
'namespace': info.entity.username || info.entity.name
|
|
};
|
|
|
|
ApiService.takeOwnership(null, params).then(function () {
|
|
callback(true);
|
|
$location.path('/organization/' + params.namespace);
|
|
}, errorDisplay)
|
|
};
|
|
|
|
$scope.checkStatus = function() {
|
|
ContainerService.checkStatus(function(resp) {
|
|
$('#restartingContainerModal').modal('hide');
|
|
$scope.configStatus = resp['status'];
|
|
$scope.requiresRestart = resp['requires_restart'];
|
|
$scope.configProviderId = resp['provider_id'];
|
|
|
|
if ($scope.configStatus == 'ready') {
|
|
$scope.currentConfig = null;
|
|
$scope.loadUsers();
|
|
} else {
|
|
var message = "Installation of this product has not yet been completed." +
|
|
"<br><br>Please read the " +
|
|
"<a href='https://coreos.com/docs/enterprise-registry/initial-setup/'>" +
|
|
"Setup Guide</a>";
|
|
|
|
var title = "Installation Incomplete";
|
|
CoreDialog.fatal(title, message);
|
|
}
|
|
}, $scope.currentConfig);
|
|
};
|
|
|
|
// Load the initial status.
|
|
$scope.checkStatus();
|
|
$scope.$watch('options.predicate', sortOrgs);
|
|
$scope.$watch('options.reverse', sortOrgs);
|
|
$scope.$watch('options.filter', sortOrgs);
|
|
|
|
}
|
|
}());
|