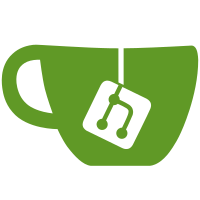
We will now cache the results of the catalog for 60s and not hit the database at all if cached
43 lines
1.6 KiB
Python
43 lines
1.6 KiB
Python
import features
|
|
|
|
from flask import jsonify
|
|
|
|
from app import model_cache
|
|
from auth.auth_context import get_authenticated_user, get_authenticated_context
|
|
from auth.registry_jwt_auth import process_registry_jwt_auth
|
|
from data.cache import cache_key
|
|
from endpoints.decorators import anon_protect
|
|
from endpoints.v2 import v2_bp, paginate
|
|
from endpoints.v2.models_interface import Repository
|
|
from endpoints.v2.models_pre_oci import data_model as model
|
|
|
|
|
|
@v2_bp.route('/_catalog', methods=['GET'])
|
|
@process_registry_jwt_auth()
|
|
@anon_protect
|
|
@paginate()
|
|
def catalog_search(start_id, limit, pagination_callback):
|
|
def _load_catalog():
|
|
include_public = bool(features.PUBLIC_CATALOG)
|
|
if not include_public and not get_authenticated_user():
|
|
return []
|
|
|
|
username = get_authenticated_user().username if get_authenticated_user() else None
|
|
if username and not get_authenticated_user().enabled:
|
|
return []
|
|
|
|
repos = model.get_visible_repositories(username, start_id, limit, include_public=include_public)
|
|
return [repo._asdict() for repo in repos]
|
|
|
|
context_key = get_authenticated_context().unique_key if get_authenticated_context() else None
|
|
catalog_cache_key = cache_key.for_catalog_page(context_key, start_id, limit)
|
|
visible_repositories = [Repository(**repo_dict) for repo_dict
|
|
in model_cache.retrieve(catalog_cache_key, _load_catalog)]
|
|
|
|
response = jsonify({
|
|
'repositories': ['%s/%s' % (repo.namespace_name, repo.name)
|
|
for repo in visible_repositories][0:limit],
|
|
})
|
|
|
|
pagination_callback(visible_repositories, response)
|
|
return response
|