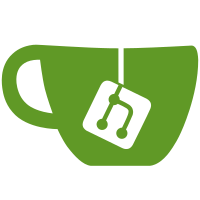
The FakeSecurityScanner mocks out all calls that Quay is expected to make to the security scanner API, and returns faked data that can be adjusted by the calling test case
86 lines
2.2 KiB
Python
86 lines
2.2 KiB
Python
import os
|
|
|
|
from datetime import datetime, timedelta
|
|
from tempfile import NamedTemporaryFile
|
|
|
|
from config import DefaultConfig
|
|
|
|
|
|
class FakeTransaction(object):
|
|
def __enter__(self):
|
|
return self
|
|
|
|
def __exit__(self, exc_type, value, traceback):
|
|
pass
|
|
|
|
|
|
TEST_DB_FILE = NamedTemporaryFile(delete=True)
|
|
|
|
|
|
class TestConfig(DefaultConfig):
|
|
TESTING = True
|
|
SECRET_KEY = 'a36c9d7d-25a9-4d3f-a586-3d2f8dc40a83'
|
|
BILLING_TYPE = 'FakeStripe'
|
|
|
|
TEST_DB_FILE = TEST_DB_FILE
|
|
DB_URI = os.environ.get('TEST_DATABASE_URI', 'sqlite:///{0}'.format(TEST_DB_FILE.name))
|
|
DB_CONNECTION_ARGS = {
|
|
'threadlocals': True,
|
|
'autorollback': True,
|
|
}
|
|
|
|
@staticmethod
|
|
def create_transaction(db):
|
|
return FakeTransaction()
|
|
|
|
DB_TRANSACTION_FACTORY = create_transaction
|
|
|
|
DISTRIBUTED_STORAGE_CONFIG = {'local_us': ['FakeStorage', {}], 'local_eu': ['FakeStorage', {}]}
|
|
DISTRIBUTED_STORAGE_PREFERENCE = ['local_us']
|
|
|
|
BUILDLOGS_MODULE_AND_CLASS = ('test.testlogs', 'testlogs.TestBuildLogs')
|
|
BUILDLOGS_OPTIONS = ['devtable', 'building', 'deadbeef-dead-beef-dead-beefdeadbeef', False]
|
|
|
|
USERFILES_LOCATION = 'local_us'
|
|
|
|
FEATURE_SUPER_USERS = True
|
|
FEATURE_BILLING = True
|
|
FEATURE_MAILING = True
|
|
SUPER_USERS = ['devtable']
|
|
|
|
LICENSE_USER_LIMIT = 500
|
|
LICENSE_EXPIRATION = datetime.now() + timedelta(weeks=520)
|
|
LICENSE_EXPIRATION_WARNING = datetime.now() + timedelta(weeks=520)
|
|
|
|
FEATURE_GITHUB_BUILD = True
|
|
FEATURE_BITTORRENT = True
|
|
FEATURE_ACI_CONVERSION = True
|
|
|
|
CLOUDWATCH_NAMESPACE = None
|
|
|
|
FEATURE_SECURITY_SCANNER = True
|
|
FEATURE_SECURITY_NOTIFICATIONS = True
|
|
SECURITY_SCANNER_ENDPOINT = 'http://fakesecurityscanner/'
|
|
SECURITY_SCANNER_API_VERSION = 'v1'
|
|
SECURITY_SCANNER_ENGINE_VERSION_TARGET = 1
|
|
SECURITY_SCANNER_API_TIMEOUT_SECONDS = 1
|
|
|
|
SIGNING_ENGINE = 'gpg2'
|
|
|
|
GPG2_PRIVATE_KEY_NAME = 'EEB32221'
|
|
GPG2_PRIVATE_KEY_FILENAME = 'test/data/signing-private.gpg'
|
|
GPG2_PUBLIC_KEY_FILENAME = 'test/data/signing-public.gpg'
|
|
|
|
INSTANCE_SERVICE_KEY_KID_LOCATION = 'test/data/test.kid'
|
|
INSTANCE_SERVICE_KEY_LOCATION = 'test/data/test.pem'
|
|
|
|
PROMETHEUS_AGGREGATOR_URL = None
|
|
|
|
FEATURE_GITHUB_LOGIN = True
|
|
FEATURE_GOOGLE_LOGIN = True
|
|
FEATURE_DEX_LOGIN = True
|
|
|
|
DEX_LOGIN_CONFIG = {
|
|
'CLIENT_ID': 'someclientid',
|
|
'OIDC_SERVER': 'https://oidcserver/',
|
|
}
|