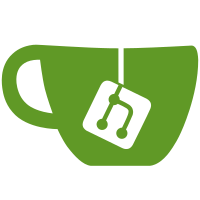
This adds additional required properties and methods to the Docker schema interface to allow us to treat both schema1 and schema2 manifests and lists logically equivalent from the OCI mode perspective
76 lines
2.4 KiB
Python
76 lines
2.4 KiB
Python
from abc import ABCMeta, abstractproperty, abstractmethod
|
|
from six import add_metaclass
|
|
|
|
@add_metaclass(ABCMeta)
|
|
class ManifestInterface(object):
|
|
""" Defines the interface for the various manifests types supported. """
|
|
@abstractproperty
|
|
def digest(self):
|
|
""" The digest of the manifest, including type prefix. """
|
|
pass
|
|
|
|
@abstractproperty
|
|
def media_type(self):
|
|
""" The media type of the schema. """
|
|
pass
|
|
|
|
@abstractproperty
|
|
def manifest_dict(self):
|
|
""" Returns the manifest as a dictionary ready to be serialized to JSON. """
|
|
pass
|
|
|
|
@abstractproperty
|
|
def bytes(self):
|
|
""" Returns the bytes of the manifest. """
|
|
pass
|
|
|
|
@abstractproperty
|
|
def layers(self):
|
|
""" Returns the layers of this manifest, from base to leaf or None if none. """
|
|
pass
|
|
|
|
@abstractproperty
|
|
def leaf_layer_v1_image_id(self):
|
|
""" Returns the Docker V1 image ID for the leaf (top) layer, if any, or None if none. """
|
|
pass
|
|
|
|
@abstractproperty
|
|
def legacy_image_ids(self):
|
|
""" Returns the Docker V1 image IDs for the layers of this manifest or None if not applicable.
|
|
"""
|
|
pass
|
|
|
|
@abstractproperty
|
|
def blob_digests(self):
|
|
""" Returns an iterator over all the blob digests referenced by this manifest,
|
|
from base to leaf. The blob digests are strings with prefixes. For manifests that reference
|
|
config as a blob, the blob will be included here.
|
|
"""
|
|
|
|
@abstractmethod
|
|
def child_manifests(self, lookup_manifest_fn):
|
|
""" Returns an iterator of all manifests that live under this manifest, if any or None if none.
|
|
The lookup_manifest_fn is a function that, when given a blob content SHA, returns the
|
|
contents of that blob in storage if any or None if none.
|
|
"""
|
|
|
|
@abstractmethod
|
|
def get_manifest_labels(self, lookup_config_fn):
|
|
""" Returns a dictionary of all the labels defined inside this manifest or None if none. """
|
|
pass
|
|
|
|
@abstractmethod
|
|
def unsigned(self):
|
|
""" Returns an unsigned version of this manifest. """
|
|
|
|
@abstractmethod
|
|
def generate_legacy_layers(self, images_map, lookup_config_fn):
|
|
"""
|
|
Rewrites Docker v1 image IDs and returns a generator of DockerV1Metadata.
|
|
|
|
If Docker gives us a layer with a v1 image ID that already points to existing
|
|
content, but the checksums don't match, then we need to rewrite the image ID
|
|
to something new in order to ensure consistency.
|
|
|
|
Returns None if there are no legacy images associated with the manifest.
|
|
"""
|