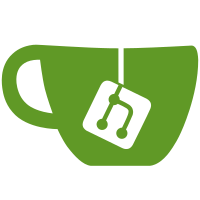
This removes the checksum backfill, removes the migration that runs the backfills, and defaults the security scan feature off.
261 lines
7.7 KiB
Python
261 lines
7.7 KiB
Python
import requests
|
|
import os.path
|
|
|
|
from data.buildlogs import BuildLogs
|
|
from data.userevent import UserEventBuilder
|
|
|
|
|
|
def build_requests_session():
|
|
sess = requests.Session()
|
|
adapter = requests.adapters.HTTPAdapter(pool_connections=100,
|
|
pool_maxsize=100)
|
|
sess.mount('http://', adapter)
|
|
sess.mount('https://', adapter)
|
|
return sess
|
|
|
|
|
|
# The set of configuration key names that will be accessible in the client. Since these
|
|
# values are sent to the frontend, DO NOT PLACE ANY SECRETS OR KEYS in this list.
|
|
CLIENT_WHITELIST = ['SERVER_HOSTNAME', 'PREFERRED_URL_SCHEME', 'MIXPANEL_KEY',
|
|
'STRIPE_PUBLISHABLE_KEY', 'ENTERPRISE_LOGO_URL', 'SENTRY_PUBLIC_DSN',
|
|
'AUTHENTICATION_TYPE', 'REGISTRY_TITLE', 'REGISTRY_TITLE_SHORT',
|
|
'CONTACT_INFO', 'AVATAR_KIND', 'LOCAL_OAUTH_HANDLER', 'DOCUMENTATION_LOCATION',
|
|
'DOCUMENTATION_METADATA', 'SETUP_COMPLETE']
|
|
|
|
|
|
def frontend_visible_config(config_dict):
|
|
visible_dict = {}
|
|
for name in CLIENT_WHITELIST:
|
|
if name.lower().find('secret') >= 0:
|
|
raise Exception('Cannot whitelist secrets: %s' % name)
|
|
|
|
if name in config_dict:
|
|
visible_dict[name] = config_dict.get(name, None)
|
|
|
|
return visible_dict
|
|
|
|
|
|
class DefaultConfig(object):
|
|
# Flask config
|
|
JSONIFY_PRETTYPRINT_REGULAR = False
|
|
SESSION_COOKIE_SECURE = False
|
|
|
|
LOGGING_LEVEL = 'DEBUG'
|
|
SEND_FILE_MAX_AGE_DEFAULT = 0
|
|
POPULATE_DB_TEST_DATA = True
|
|
PREFERRED_URL_SCHEME = 'http'
|
|
SERVER_HOSTNAME = 'localhost:5000'
|
|
|
|
REGISTRY_TITLE = 'Quay Enterprise'
|
|
REGISTRY_TITLE_SHORT = 'Quay Enterprise'
|
|
|
|
CONTACT_INFO = [
|
|
'mailto:support@quay.io',
|
|
'irc://chat.freenode.net:6665/quayio',
|
|
'tel:+1-888-930-3475',
|
|
'https://twitter.com/quayio',
|
|
]
|
|
|
|
# Mail config
|
|
MAIL_SERVER = ''
|
|
MAIL_USE_TLS = True
|
|
MAIL_PORT = 587
|
|
MAIL_USERNAME = None
|
|
MAIL_PASSWORD = None
|
|
MAIL_DEFAULT_SENDER = 'support@quay.io'
|
|
MAIL_FAIL_SILENTLY = False
|
|
TESTING = True
|
|
|
|
# DB config
|
|
DB_URI = 'sqlite:///test/data/test.db'
|
|
DB_CONNECTION_ARGS = {
|
|
'threadlocals': True,
|
|
'autorollback': True,
|
|
}
|
|
|
|
@staticmethod
|
|
def create_transaction(db):
|
|
return db.transaction()
|
|
|
|
DB_TRANSACTION_FACTORY = create_transaction
|
|
|
|
# If true, CDN URLs will be used for our external dependencies, rather than the local
|
|
# copies.
|
|
USE_CDN = True
|
|
|
|
# Authentication
|
|
AUTHENTICATION_TYPE = 'Database'
|
|
|
|
# Build logs
|
|
BUILDLOGS_REDIS = {'host': 'localhost'}
|
|
BUILDLOGS_OPTIONS = []
|
|
|
|
# Real-time user events
|
|
USER_EVENTS_REDIS = {'host': 'localhost'}
|
|
|
|
# Stripe config
|
|
BILLING_TYPE = 'FakeStripe'
|
|
|
|
# Analytics
|
|
ANALYTICS_TYPE = 'FakeAnalytics'
|
|
|
|
# Build Queue Metrics
|
|
QUEUE_METRICS_TYPE = 'Null'
|
|
|
|
# Exception logging
|
|
EXCEPTION_LOG_TYPE = 'FakeSentry'
|
|
SENTRY_DSN = None
|
|
SENTRY_PUBLIC_DSN = None
|
|
|
|
# Github Config
|
|
GITHUB_LOGIN_CONFIG = None
|
|
GITHUB_TRIGGER_CONFIG = None
|
|
|
|
# Google Config.
|
|
GOOGLE_LOGIN_CONFIG = None
|
|
|
|
# Bitbucket Config.
|
|
BITBUCKET_TRIGGER_CONFIG = None
|
|
|
|
# Requests based HTTP client with a large request pool
|
|
HTTPCLIENT = build_requests_session()
|
|
|
|
# Status tag config
|
|
STATUS_TAGS = {}
|
|
for tag_name in ['building', 'failed', 'none', 'ready']:
|
|
tag_path = os.path.join('buildstatus', tag_name + '.svg')
|
|
with open(tag_path) as tag_svg:
|
|
STATUS_TAGS[tag_name] = tag_svg.read()
|
|
|
|
NOTIFICATION_QUEUE_NAME = 'notification'
|
|
DIFFS_QUEUE_NAME = 'imagediff'
|
|
DOCKERFILE_BUILD_QUEUE_NAME = 'dockerfilebuild'
|
|
REPLICATION_QUEUE_NAME = 'imagestoragereplication'
|
|
|
|
# Super user config. Note: This MUST BE an empty list for the default config.
|
|
SUPER_USERS = []
|
|
|
|
# Feature Flag: Whether super users are supported.
|
|
FEATURE_SUPER_USERS = True
|
|
|
|
# Feature Flag: Whether to allow anonymous users to browse and pull public repositories.
|
|
FEATURE_ANONYMOUS_ACCESS = True
|
|
|
|
# Feature Flag: Whether billing is required.
|
|
FEATURE_BILLING = False
|
|
|
|
# Feature Flag: Whether user accounts automatically have usage log access.
|
|
FEATURE_USER_LOG_ACCESS = False
|
|
|
|
# Feature Flag: Whether GitHub login is supported.
|
|
FEATURE_GITHUB_LOGIN = False
|
|
|
|
# Feature Flag: Whether Google login is supported.
|
|
FEATURE_GOOGLE_LOGIN = False
|
|
|
|
# Feature Flag: Whther Dex login is supported.
|
|
FEATURE_DEX_LOGIN = False
|
|
|
|
# Feature flag, whether to enable olark chat
|
|
FEATURE_OLARK_CHAT = False
|
|
|
|
# Feature Flag: Whether to support GitHub build triggers.
|
|
FEATURE_GITHUB_BUILD = False
|
|
|
|
# Feature Flag: Whether to support Bitbucket build triggers.
|
|
FEATURE_BITBUCKET_BUILD = False
|
|
|
|
# Feature Flag: Whether to support GitLab build triggers.
|
|
FEATURE_GITLAB_BUILD = False
|
|
|
|
# Feature Flag: Dockerfile build support.
|
|
FEATURE_BUILD_SUPPORT = True
|
|
|
|
# Feature Flag: Whether emails are enabled.
|
|
FEATURE_MAILING = True
|
|
|
|
# Feature Flag: Whether users can be created (by non-super users).
|
|
FEATURE_USER_CREATION = True
|
|
|
|
# Feature Flag: Whether users can be renamed
|
|
FEATURE_USER_RENAME = False
|
|
|
|
# Feature Flag: Whether non-encrypted passwords (as opposed to encrypted tokens) can be used for
|
|
# basic auth.
|
|
FEATURE_REQUIRE_ENCRYPTED_BASIC_AUTH = False
|
|
|
|
# Feature Flag: Whether to automatically replicate between storage engines.
|
|
FEATURE_STORAGE_REPLICATION = False
|
|
|
|
# Feature Flag: Whether users can directly login to the UI.
|
|
FEATURE_DIRECT_LOGIN = True
|
|
|
|
BUILD_MANAGER = ('enterprise', {})
|
|
|
|
DISTRIBUTED_STORAGE_CONFIG = {
|
|
'local_eu': ['LocalStorage', {'storage_path': 'test/data/registry/eu'}],
|
|
'local_us': ['LocalStorage', {'storage_path': 'test/data/registry/us'}],
|
|
}
|
|
|
|
DISTRIBUTED_STORAGE_PREFERENCE = ['local_us']
|
|
DISTRIBUTED_STORAGE_DEFAULT_LOCATIONS = ['local_us']
|
|
|
|
# Health checker.
|
|
HEALTH_CHECKER = ('LocalHealthCheck', {})
|
|
|
|
# Userfiles
|
|
USERFILES_LOCATION = 'local_us'
|
|
USERFILES_PATH = 'userfiles/'
|
|
|
|
# Build logs archive
|
|
LOG_ARCHIVE_LOCATION = 'local_us'
|
|
LOG_ARCHIVE_PATH = 'logarchive/'
|
|
|
|
# For enterprise:
|
|
MAXIMUM_REPOSITORY_USAGE = 20
|
|
|
|
# System logs.
|
|
SYSTEM_LOGS_PATH = "/var/log/"
|
|
SYSTEM_LOGS_FILE = "/var/log/syslog"
|
|
SYSTEM_SERVICES_PATH = "conf/init/service/"
|
|
|
|
# Services that should not be shown in the logs view.
|
|
SYSTEM_SERVICE_BLACKLIST = []
|
|
|
|
# Temporary tag expiration in seconds, this may actually be longer based on GC policy
|
|
PUSH_TEMP_TAG_EXPIRATION_SEC = 60 * 60 # One hour per layer
|
|
|
|
# Signed registry grant token expiration in seconds
|
|
SIGNED_GRANT_EXPIRATION_SEC = 60 * 60 * 24 # One day to complete a push/pull
|
|
|
|
# Registry v2 JWT Auth config
|
|
JWT_AUTH_MAX_FRESH_S = 60 * 5 # At most the JWT can be signed for 300s in the future
|
|
JWT_AUTH_CERTIFICATE_PATH = 'conf/selfsigned/jwt.crt'
|
|
JWT_AUTH_PRIVATE_KEY_PATH = 'conf/selfsigned/jwt.key.insecure'
|
|
|
|
# The URL endpoint to which we redirect OAuth when generating a token locally.
|
|
LOCAL_OAUTH_HANDLER = '/oauth/localapp'
|
|
|
|
# The various avatar background colors.
|
|
AVATAR_KIND = 'local'
|
|
AVATAR_COLORS = ['#969696', '#aec7e8', '#ff7f0e', '#ffbb78', '#2ca02c', '#98df8a', '#d62728',
|
|
'#ff9896', '#9467bd', '#c5b0d5', '#8c564b', '#c49c94', '#e377c2', '#f7b6d2',
|
|
'#7f7f7f', '#c7c7c7', '#bcbd22', '#1f77b4', '#17becf', '#9edae5', '#393b79',
|
|
'#5254a3', '#6b6ecf', '#9c9ede', '#9ecae1', '#31a354', '#b5cf6b', '#a1d99b',
|
|
'#8c6d31', '#ad494a', '#e7ba52', '#a55194']
|
|
|
|
# The location of the Quay documentation.
|
|
DOCUMENTATION_LOCATION = 'http://docs.quay.io'
|
|
DOCUMENTATION_METADATA = 'https://coreos.github.io/quay-docs/search.json'
|
|
|
|
# Experiment: Async garbage collection
|
|
EXP_ASYNC_GARBAGE_COLLECTION = []
|
|
|
|
# Security scanner
|
|
FEATURE_SECURITY_SCANNER = False
|
|
SECURITY_SCANNER = {
|
|
'ENDPOINT': 'http://192.168.99.100:6060',
|
|
'ENGINE_VERSION_TARGET': 1,
|
|
'API_VERSION': 'v1',
|
|
'API_TIMEOUT_SECONDS': 10,
|
|
}
|