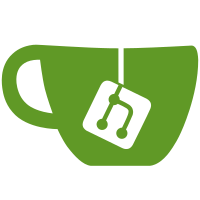
Allows us to have a new config provider for each setup, with no overlap of the directories used, and automatic cleanup of those directories.
30 lines
1.3 KiB
Python
30 lines
1.3 KiB
Python
import os
|
|
from backports.tempfile import TemporaryDirectory
|
|
|
|
from config_app.config_util.config.fileprovider import FileConfigProvider
|
|
|
|
class TransientDirectoryProvider(FileConfigProvider):
|
|
""" Implementation of the config provider that reads and writes the data
|
|
from/to the file system, only using temporary directories,
|
|
deleting old dirs and creating new ones as requested.
|
|
"""
|
|
def __init__(self, config_volume, yaml_filename, py_filename):
|
|
# Create a temp directory that will be cleaned up when we change the config path
|
|
# This should ensure we have no "pollution" of different configs:
|
|
# no uploaded config should ever affect subsequent config modifications/creations
|
|
temp_dir = TemporaryDirectory()
|
|
self.temp_dir = temp_dir
|
|
super(TransientDirectoryProvider, self).__init__(temp_dir.name, yaml_filename, py_filename)
|
|
|
|
def new_config_dir(self):
|
|
"""
|
|
Update the path with a new temporary directory, deleting the old one in the process
|
|
"""
|
|
temp_dir = TemporaryDirectory()
|
|
|
|
self.config_volume = temp_dir.name
|
|
self.temp_dir = temp_dir
|
|
self.yaml_path = os.path.join(temp_dir.name, self.yaml_filename)
|
|
|
|
def get_config_dir_path(self):
|
|
return self.config_volume
|