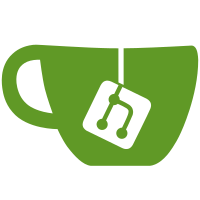
We move all the auth handling, serialization and deserialization into a new AuthContext interface, and then standardize a registration model for handling of specific auth context types (user, robot, token, etc).
26 lines
999 B
Python
26 lines
999 B
Python
import features
|
|
|
|
from flask import jsonify
|
|
|
|
from auth.auth_context import get_authenticated_user
|
|
from auth.registry_jwt_auth import process_registry_jwt_auth
|
|
from endpoints.decorators import anon_protect
|
|
from endpoints.v2 import v2_bp, paginate
|
|
from endpoints.v2.models_pre_oci import data_model as model
|
|
|
|
|
|
@v2_bp.route('/_catalog', methods=['GET'])
|
|
@process_registry_jwt_auth()
|
|
@anon_protect
|
|
@paginate()
|
|
def catalog_search(limit, offset, pagination_callback):
|
|
username = get_authenticated_user().username if get_authenticated_user() else None
|
|
include_public = bool(features.PUBLIC_CATALOG)
|
|
visible_repositories = model.get_visible_repositories(username, limit + 1, offset,
|
|
include_public=include_public)
|
|
response = jsonify({
|
|
'repositories': ['%s/%s' % (repo.namespace_name, repo.name)
|
|
for repo in visible_repositories][0:limit],})
|
|
|
|
pagination_callback(len(visible_repositories), response)
|
|
return response
|