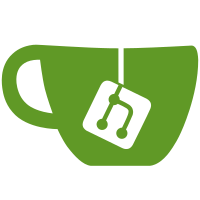
This allows the repositories to be selected in the UI, if we are unsure whether the user has permission. Since gitlab will do the check anyway, this is safe, although not a great user experience if they chose an invalid repository, but we can't really do much about that.
114 lines
3.1 KiB
Python
114 lines
3.1 KiB
Python
import json
|
|
import pytest
|
|
|
|
from mock import Mock
|
|
|
|
from buildtrigger.test.gitlabmock import get_gitlab_trigger, get_mock_gitlab
|
|
from buildtrigger.triggerutil import (SkipRequestException, ValidationRequestException,
|
|
InvalidPayloadException)
|
|
from endpoints.building import PreparedBuild
|
|
from util.morecollections import AttrDict
|
|
|
|
@pytest.fixture
|
|
def gitlab_trigger():
|
|
return get_gitlab_trigger()
|
|
|
|
|
|
def test_list_build_subdirs(gitlab_trigger):
|
|
assert gitlab_trigger.list_build_subdirs() == ['/Dockerfile']
|
|
|
|
|
|
@pytest.mark.parametrize('dockerfile_path, contents', [
|
|
('/Dockerfile', 'hello world'),
|
|
('somesubdir/Dockerfile', 'hi universe'),
|
|
('unknownpath', None),
|
|
])
|
|
def test_load_dockerfile_contents(dockerfile_path, contents):
|
|
trigger = get_gitlab_trigger(dockerfile_path)
|
|
assert trigger.load_dockerfile_contents() == contents
|
|
|
|
|
|
@pytest.mark.parametrize('email, expected_response', [
|
|
('unknown@email.com', None),
|
|
('knownuser', {'username': 'knownuser', 'html_url': 'https://bitbucket.org/knownuser',
|
|
'avatar_url': 'avatarurl'}),
|
|
])
|
|
def test_lookup_user(email, expected_response, gitlab_trigger):
|
|
assert gitlab_trigger.lookup_user(email) == expected_response
|
|
|
|
|
|
def test_null_permissions(gitlab_trigger):
|
|
gitlab_trigger._get_authorized_client = get_mock_gitlab(with_nulls=True)
|
|
sources = gitlab_trigger.list_build_sources_for_namespace('someorg')
|
|
source = sources[0]
|
|
assert source['has_admin_permissions']
|
|
|
|
|
|
def test_null_avatar(gitlab_trigger):
|
|
gitlab_trigger._get_authorized_client = get_mock_gitlab(with_nulls=True)
|
|
namespace_data = gitlab_trigger.list_build_source_namespaces()
|
|
expected = {
|
|
'avatar_url': None,
|
|
'personal': False,
|
|
'title': 'someorg',
|
|
'url': 'https://bitbucket.org/someorg',
|
|
'score': 1,
|
|
'id': 'someorg',
|
|
}
|
|
|
|
assert namespace_data == [expected]
|
|
|
|
|
|
@pytest.mark.parametrize('payload, expected_error, expected_message', [
|
|
('{}', InvalidPayloadException, ''),
|
|
|
|
# Valid payload:
|
|
('''{
|
|
"object_kind": "push",
|
|
"ref": "refs/heads/master",
|
|
"checkout_sha": "aaaaaaa",
|
|
"repository": {
|
|
"git_ssh_url": "foobar"
|
|
},
|
|
"commits": [
|
|
{
|
|
"id": "aaaaaaa",
|
|
"url": "someurl",
|
|
"message": "hello there!",
|
|
"timestamp": "now"
|
|
}
|
|
]
|
|
}''', None, None),
|
|
|
|
# Skip message:
|
|
('''{
|
|
"object_kind": "push",
|
|
"ref": "refs/heads/master",
|
|
"checkout_sha": "aaaaaaa",
|
|
"repository": {
|
|
"git_ssh_url": "foobar"
|
|
},
|
|
"commits": [
|
|
{
|
|
"id": "aaaaaaa",
|
|
"url": "someurl",
|
|
"message": "[skip build] hello there!",
|
|
"timestamp": "now"
|
|
}
|
|
]
|
|
}''', SkipRequestException, ''),
|
|
])
|
|
def test_handle_trigger_request(gitlab_trigger, payload, expected_error, expected_message):
|
|
def get_payload():
|
|
return json.loads(payload)
|
|
|
|
request = AttrDict(dict(get_json=get_payload))
|
|
|
|
if expected_error is not None:
|
|
with pytest.raises(expected_error) as ipe:
|
|
gitlab_trigger.handle_trigger_request(request)
|
|
assert ipe.value.message == expected_message
|
|
else:
|
|
assert isinstance(gitlab_trigger.handle_trigger_request(request), PreparedBuild)
|
|
|
|
|