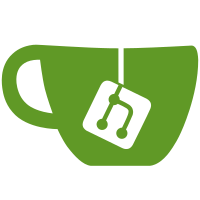
Should prevent a customer from accidentally saving a config that violates their license Fixes https://jira.coreos.com/browse/QS-97
19 lines
778 B
Python
19 lines
778 B
Python
from app import config_provider
|
|
from util.config.validators import BaseValidator, ConfigValidationException
|
|
from util.license import LicenseDecodeError, EntitlementStatus
|
|
|
|
class LicenseValidator(BaseValidator):
|
|
name = "license"
|
|
|
|
@classmethod
|
|
def validate(cls, config, user, user_password):
|
|
try:
|
|
decoded_license = config_provider.get_license()
|
|
except LicenseDecodeError as le:
|
|
raise ConfigValidationException('Could not decode license: %s' % le.message)
|
|
|
|
results = decoded_license.validate(config)
|
|
all_met = all(result.is_met() for result in results)
|
|
if not all_met:
|
|
reason = [result.description() for result in results if not result.is_met()]
|
|
raise ConfigValidationException('License does not match configuration: %s' % reason)
|