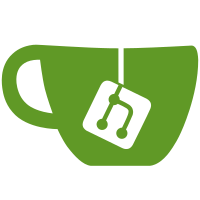
This isolates the CloudWatch sending thread to it's own package where it can be shared among any other packages that want to asynchronously send metrics to CloudWatch.
51 lines
1.4 KiB
Python
51 lines
1.4 KiB
Python
import logging
|
|
import boto
|
|
|
|
from Queue import Queue
|
|
from threading import Thread
|
|
|
|
|
|
logger = logging.getLogger(__name__)
|
|
queue = None
|
|
|
|
def get_queue(app):
|
|
"""
|
|
Returns a queue to the CloudWatchSender. If a queue/sender do not exist, creates them.
|
|
"""
|
|
if queue is None:
|
|
global queue
|
|
access_key = app.config.get('QUEUE_METRICS_AWS_ACCESS_KEY')
|
|
secret_key = app.config.get('QUEUE_METRICS_AWS_SECRET_KEY')
|
|
queue = Queue()
|
|
sender = CloudWatchSender(queue, access_key, secret_key)
|
|
sender.start()
|
|
else:
|
|
return queue
|
|
|
|
|
|
class CloudWatchSender(Thread):
|
|
"""
|
|
CloudWatchSender loops indefinitely and pulls metrics off of a queue then sends it to CloudWatch.
|
|
"""
|
|
def __init__(self, request_queue, aws_access_key, aws_secret_key):
|
|
Thread.__init__(self)
|
|
self.daemon = True
|
|
|
|
self._aws_access_key = aws_access_key
|
|
self._aws_secret_key = aws_secret_key
|
|
self._put_metrics_queue = request_queue
|
|
|
|
def run(self):
|
|
try:
|
|
logger.debug('Starting CloudWatch sender process.')
|
|
connection = boto.connect_cloudwatch(self._aws_access_key, self._aws_secret_key)
|
|
except:
|
|
logger.exception('Failed to connect to CloudWatch.')
|
|
|
|
while True:
|
|
put_metric_args, kwargs = self._put_metrics_queue.get()
|
|
logger.debug('Got queued put metrics request.')
|
|
try:
|
|
connection.put_metric_data(*put_metric_args, **kwargs)
|
|
except:
|
|
logger.exception('Failed to write to CloudWatch')
|