forked from mirrors/homebox
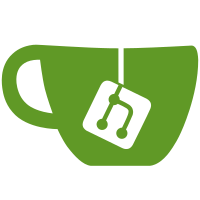
* change /content/ -> /homebox/ * add cache to code generators * update env variables to set data storage * update env variables * set env variables in prod container * implement attachment post route (WIP) * get attachment endpoint * attachment download * implement string utilities lib * implement generic drop zone * use explicit truncate * remove clean dir * drop strings composable for lib * update item types and add attachments * add attachment API * implement service context * consolidate API code * implement editing attachments * implement upload limit configuration * improve error handling * add docs for max upload size * fix test cases
94 lines
1.6 KiB
Go
94 lines
1.6 KiB
Go
package pathlib
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
func Test_hasConflict(t *testing.T) {
|
|
type args struct {
|
|
path string
|
|
neighbors []string
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want bool
|
|
}{
|
|
{
|
|
name: "no conflict",
|
|
args: args{
|
|
path: "foo",
|
|
neighbors: []string{"bar", "baz"},
|
|
},
|
|
want: false,
|
|
},
|
|
{
|
|
name: "conflict",
|
|
args: args{
|
|
path: "foo",
|
|
neighbors: []string{"bar", "foo"},
|
|
},
|
|
want: true,
|
|
},
|
|
{
|
|
name: "conflict with different case",
|
|
args: args{
|
|
path: "foo",
|
|
neighbors: []string{"bar", "Foo"},
|
|
},
|
|
want: true,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if got := hasConflict(tt.args.path, tt.args.neighbors); got != tt.want {
|
|
t.Errorf("hasConflict() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestSafePath(t *testing.T) {
|
|
// override dirReader
|
|
dirReader = func(name string) []string {
|
|
return []string{"bar.pdf", "bar (1).pdf", "bar (2).pdf"}
|
|
}
|
|
|
|
type args struct {
|
|
path string
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want string
|
|
}{
|
|
{
|
|
name: "no conflict",
|
|
args: args{
|
|
path: "/foo/foo.pdf",
|
|
},
|
|
want: "/foo/foo.pdf",
|
|
},
|
|
{
|
|
name: "conflict",
|
|
args: args{
|
|
path: "/foo/bar.pdf",
|
|
},
|
|
want: "/foo/bar (3).pdf",
|
|
},
|
|
{
|
|
name: "conflict with different case",
|
|
args: args{
|
|
path: "/foo/BAR.pdf",
|
|
},
|
|
want: "/foo/BAR (3).pdf",
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if got := Safe(tt.args.path); got != tt.want {
|
|
t.Errorf("SafePath() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|