forked from mirrors/homebox
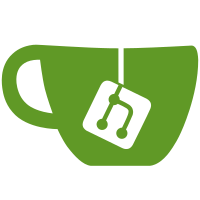
* format readme * update logo * format html * add logo to docs * repository for document and document tokens * add attachments type and repository * autogenerate types via scripts * use autogenerated types * attachment type updates * add insured and quantity fields for items * implement HasID interface for entities * implement label updates for items * implement service update method * WIP item update client side actions * check err on attachment * finish types for basic items editor * remove unused var * house keeping
30 lines
1 KiB
TypeScript
30 lines
1 KiB
TypeScript
import { describe, expect, test } from "vitest";
|
|
import { hasKey, parseDate } from "./base-api";
|
|
|
|
describe("hasKey works as expected", () => {
|
|
test("hasKey returns true if the key exists", () => {
|
|
const obj = { createdAt: "2021-01-01" };
|
|
expect(hasKey(obj, "createdAt")).toBe(true);
|
|
});
|
|
|
|
test("hasKey returns false if the key does not exist", () => {
|
|
const obj = { createdAt: "2021-01-01" };
|
|
expect(hasKey(obj, "updatedAt")).toBe(false);
|
|
});
|
|
});
|
|
|
|
describe("parseDate should work as expected", () => {
|
|
test("parseDate should set defaults", () => {
|
|
const obj = { createdAt: "2021-01-01", updatedAt: "2021-01-01" };
|
|
const result = parseDate(obj);
|
|
expect(result.createdAt).toBeInstanceOf(Date);
|
|
expect(result.updatedAt).toBeInstanceOf(Date);
|
|
});
|
|
|
|
test("parseDate should set passed in types", () => {
|
|
const obj = { key1: "2021-01-01", key2: "2021-01-01" };
|
|
const result = parseDate(obj, ["key1", "key2"]);
|
|
expect(result.key1).toBeInstanceOf(Date);
|
|
expect(result.key2).toBeInstanceOf(Date);
|
|
});
|
|
});
|