forked from mirrors/homebox
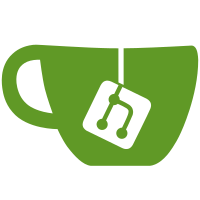
* implement password score UI and functions * update strings tests to use `test`instead of `it` * update typing * refactor login/register UI+Logic * fix width on switches to properly display * fetch and store self in store * (WIP) unify card styles * update labels page * bump nuxt * use form area * use text area for description * unify confirm API * unify UI around pages * change header background height
37 lines
942 B
TypeScript
37 lines
942 B
TypeScript
import { BaseAPI, route } from "./base";
|
|
import { ItemsApi } from "./classes/items";
|
|
import { LabelsApi } from "./classes/labels";
|
|
import { LocationsApi } from "./classes/locations";
|
|
import { UserOut } from "./types/data-contracts";
|
|
import { Requests } from "~~/lib/requests";
|
|
|
|
export type Result<T> = {
|
|
item: T;
|
|
};
|
|
|
|
export class UserApi extends BaseAPI {
|
|
locations: LocationsApi;
|
|
labels: LabelsApi;
|
|
items: ItemsApi;
|
|
constructor(requests: Requests) {
|
|
super(requests);
|
|
|
|
this.locations = new LocationsApi(requests);
|
|
this.labels = new LabelsApi(requests);
|
|
this.items = new ItemsApi(requests);
|
|
|
|
Object.freeze(this);
|
|
}
|
|
|
|
public self() {
|
|
return this.http.get<Result<UserOut>>({ url: route("/users/self") });
|
|
}
|
|
|
|
public logout() {
|
|
return this.http.post<object, void>({ url: route("/users/logout") });
|
|
}
|
|
|
|
public deleteAccount() {
|
|
return this.http.delete<void>({ url: route("/users/self") });
|
|
}
|
|
}
|