forked from mirrors/homebox
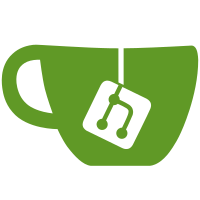
* implement custom http handler interface * implement trace_id * normalize http method spacing for consistent logs * fix failing test * fix linter errors * cleanup old dead code * more route cleanup * cleanup some inconsistent errors * update and generate code * make taskfile more consistent * update task calls * run tidy * drop `@` tag for version * use relative paths * tidy * fix auto-setting variables * update build paths * add contributing guide * tidy
25 lines
651 B
Go
25 lines
651 B
Go
package server
|
|
|
|
import (
|
|
"net/http"
|
|
)
|
|
|
|
type HandlerFunc func(w http.ResponseWriter, r *http.Request) error
|
|
|
|
func (f HandlerFunc) ServeHTTP(w http.ResponseWriter, r *http.Request) error {
|
|
return f(w, r)
|
|
}
|
|
|
|
type Handler interface {
|
|
ServeHTTP(http.ResponseWriter, *http.Request) error
|
|
}
|
|
|
|
// ToHandler converts a function to a customer implementation of the Handler interface.
|
|
// that returns an error. This wrapper around the handler function and simply
|
|
// returns the nil in all cases
|
|
func ToHandler(handler http.Handler) Handler {
|
|
return HandlerFunc(func(w http.ResponseWriter, r *http.Request) error {
|
|
handler.ServeHTTP(w, r)
|
|
return nil
|
|
})
|
|
}
|