forked from mirrors/homebox
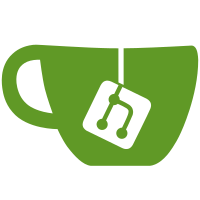
* fix potential memory leak with time.After * add new background service to manage scheduled notifications * update docs * remove old js reference * closes #278 * tidy
81 lines
1.5 KiB
Go
81 lines
1.5 KiB
Go
package services
|
|
|
|
import (
|
|
"context"
|
|
"strings"
|
|
"time"
|
|
|
|
"github.com/containrrr/shoutrrr"
|
|
"github.com/hay-kot/homebox/backend/internal/data/repo"
|
|
"github.com/hay-kot/homebox/backend/internal/data/types"
|
|
"github.com/rs/zerolog/log"
|
|
)
|
|
|
|
type BackgroundService struct {
|
|
repos *repo.AllRepos
|
|
}
|
|
|
|
func (svc *BackgroundService) SendNotifiersToday(ctx context.Context) error {
|
|
// Get All Groups
|
|
groups, err := svc.repos.Groups.GetAllGroups(ctx)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
today := types.DateFromTime(time.Now())
|
|
|
|
for i := range groups {
|
|
group := groups[i]
|
|
|
|
entries, err := svc.repos.MaintEntry.GetScheduled(ctx, group.ID, today)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if len(entries) == 0 {
|
|
log.Debug().
|
|
Str("group_name", group.Name).
|
|
Str("group_id", group.ID.String()).
|
|
Msg("No scheduled maintenance for today")
|
|
continue
|
|
}
|
|
|
|
notifiers, err := svc.repos.Notifiers.GetByGroup(ctx, group.ID)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
urls := make([]string, len(notifiers))
|
|
for i := range notifiers {
|
|
urls[i] = notifiers[i].URL
|
|
}
|
|
|
|
bldr := strings.Builder{}
|
|
|
|
bldr.WriteString("Homebox Maintenance for (")
|
|
bldr.WriteString(today.String())
|
|
bldr.WriteString("):\n")
|
|
|
|
for i := range entries {
|
|
entry := entries[i]
|
|
bldr.WriteString(" - ")
|
|
bldr.WriteString(entry.Name)
|
|
bldr.WriteString("\n")
|
|
}
|
|
|
|
var sendErrs []error
|
|
for i := range urls {
|
|
err := shoutrrr.Send(urls[i], bldr.String())
|
|
|
|
if err != nil {
|
|
sendErrs = append(sendErrs, err)
|
|
}
|
|
}
|
|
|
|
if len(sendErrs) > 0 {
|
|
return sendErrs[0]
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|