forked from mirrors/homebox
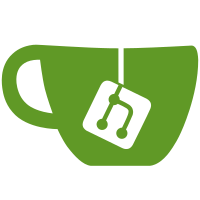
* fix session clearing on error * use singleton context to manage user state * implement remember-me functionality * fix errors * fix more errors
30 lines
788 B
TypeScript
30 lines
788 B
TypeScript
import { BaseAPI, route } from "./base";
|
|
import { ApiSummary, LoginForm, TokenResponse, UserRegistration } from "./types/data-contracts";
|
|
|
|
export type StatusResult = {
|
|
health: boolean;
|
|
versions: string[];
|
|
title: string;
|
|
message: string;
|
|
};
|
|
|
|
export class PublicApi extends BaseAPI {
|
|
public status() {
|
|
return this.http.get<ApiSummary>({ url: route("/status") });
|
|
}
|
|
|
|
public login(username: string, password: string, stayLoggedIn = false) {
|
|
return this.http.post<LoginForm, TokenResponse>({
|
|
url: route("/users/login"),
|
|
body: {
|
|
username,
|
|
password,
|
|
stayLoggedIn,
|
|
},
|
|
});
|
|
}
|
|
|
|
public register(body: UserRegistration) {
|
|
return this.http.post<UserRegistration, TokenResponse>({ url: route("/users/register"), body });
|
|
}
|
|
}
|