forked from mirrors/homebox
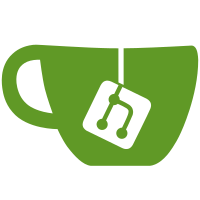
* implement password score UI and functions * update strings tests to use `test`instead of `it` * update typing * refactor login/register UI+Logic * fix width on switches to properly display * fetch and store self in store * (WIP) unify card styles * update labels page * bump nuxt * use form area * use text area for description * unify confirm API * unify UI around pages * change header background height
37 lines
811 B
TypeScript
37 lines
811 B
TypeScript
import type { ComputedRef, Ref } from "vue";
|
|
import { scorePassword } from "~~/lib/passwords";
|
|
|
|
export interface PasswordScore {
|
|
score: ComputedRef<number>;
|
|
message: ComputedRef<string>;
|
|
isValid: ComputedRef<boolean>;
|
|
}
|
|
|
|
export function usePasswordScore(pw: Ref<string>, min = 30): PasswordScore {
|
|
const score = computed(() => {
|
|
return scorePassword(pw.value) || 0;
|
|
});
|
|
|
|
const message = computed(() => {
|
|
if (score.value < 20) {
|
|
return "Very weak";
|
|
} else if (score.value < 40) {
|
|
return "Weak";
|
|
} else if (score.value < 60) {
|
|
return "Good";
|
|
} else if (score.value < 80) {
|
|
return "Strong";
|
|
}
|
|
return "Very strong";
|
|
});
|
|
|
|
const isValid = computed(() => {
|
|
return score.value >= min;
|
|
});
|
|
|
|
return {
|
|
score,
|
|
isValid,
|
|
message,
|
|
};
|
|
}
|