forked from mirrors/homebox
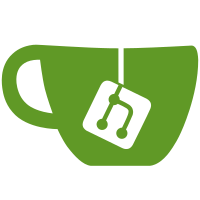
* add user profiles and theme selectors * lowercase buttons by default * basic layout * (wip) init token APIs * refactor server to support variable options * fix types * api refactor / registration tests * implement UI for url and join * remove console.logs * rename repository factory * fix upload size
45 lines
1.1 KiB
TypeScript
45 lines
1.1 KiB
TypeScript
import { defineStore } from "pinia";
|
|
import { useLocalStorage } from "@vueuse/core";
|
|
import { UserClient } from "~~/lib/api/user";
|
|
import { UserOut } from "~~/lib/api/types/data-contracts";
|
|
|
|
export const useAuthStore = defineStore("auth", {
|
|
state: () => ({
|
|
token: useLocalStorage("pinia/auth/token", ""),
|
|
expires: useLocalStorage("pinia/auth/expires", ""),
|
|
self: null as UserOut | null,
|
|
}),
|
|
getters: {
|
|
isTokenExpired: state => {
|
|
if (!state.expires) {
|
|
return true;
|
|
}
|
|
|
|
if (typeof state.expires === "string") {
|
|
return new Date(state.expires) < new Date();
|
|
}
|
|
|
|
return state.expires < new Date();
|
|
},
|
|
},
|
|
actions: {
|
|
async logout(api: UserClient) {
|
|
const result = await api.user.logout();
|
|
|
|
this.token = "";
|
|
this.expires = "";
|
|
this.self = null;
|
|
|
|
return result;
|
|
},
|
|
/**
|
|
* clearSession is used when the user cannot be logged out via the API and
|
|
* must clear it's local session, usually when a 401 is received.
|
|
*/
|
|
clearSession() {
|
|
this.token = "";
|
|
this.expires = "";
|
|
navigateTo("/");
|
|
},
|
|
},
|
|
});
|