forked from mirrors/homebox
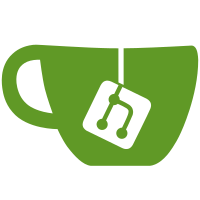
* format readme * update logo * format html * add logo to docs * repository for document and document tokens * add attachments type and repository * autogenerate types via scripts * use autogenerated types * attachment type updates * add insured and quantity fields for items * implement HasID interface for entities * implement label updates for items * implement service update method * WIP item update client side actions * check err on attachment * finish types for basic items editor * remove unused var * house keeping
47 lines
1.3 KiB
Go
47 lines
1.3 KiB
Go
package repo
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/google/uuid"
|
|
"github.com/hay-kot/content/backend/ent"
|
|
"github.com/hay-kot/content/backend/ent/document"
|
|
"github.com/hay-kot/content/backend/ent/group"
|
|
"github.com/hay-kot/content/backend/internal/types"
|
|
)
|
|
|
|
// DocumentRepository is a repository for Document entity
|
|
type DocumentRepository struct {
|
|
db *ent.Client
|
|
}
|
|
|
|
func (r *DocumentRepository) Create(ctx context.Context, gid uuid.UUID, doc types.DocumentCreate) (*ent.Document, error) {
|
|
return r.db.Document.Create().
|
|
SetGroupID(gid).
|
|
SetTitle(doc.Title).
|
|
SetPath(doc.Path).
|
|
Save(ctx)
|
|
}
|
|
|
|
func (r *DocumentRepository) GetAll(ctx context.Context, gid uuid.UUID) ([]*ent.Document, error) {
|
|
return r.db.Document.Query().
|
|
Where(document.HasGroupWith(group.ID(gid))).
|
|
All(ctx)
|
|
}
|
|
|
|
func (r *DocumentRepository) Get(ctx context.Context, id uuid.UUID) (*ent.Document, error) {
|
|
return r.db.Document.Query().
|
|
Where(document.ID(id)).
|
|
Only(ctx)
|
|
}
|
|
|
|
func (r *DocumentRepository) Update(ctx context.Context, id uuid.UUID, doc types.DocumentUpdate) (*ent.Document, error) {
|
|
return r.db.Document.UpdateOneID(id).
|
|
SetTitle(doc.Title).
|
|
SetPath(doc.Path).
|
|
Save(ctx)
|
|
}
|
|
|
|
func (r *DocumentRepository) Delete(ctx context.Context, id uuid.UUID) error {
|
|
return r.db.Document.DeleteOneID(id).Exec(ctx)
|
|
}
|