forked from mirrors/homebox
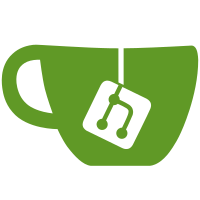
* format readme * update logo * format html * add logo to docs * repository for document and document tokens * add attachments type and repository * autogenerate types via scripts * use autogenerated types * attachment type updates * add insured and quantity fields for items * implement HasID interface for entities * implement label updates for items * implement service update method * WIP item update client side actions * check err on attachment * finish types for basic items editor * remove unused var * house keeping
41 lines
1.1 KiB
Go
41 lines
1.1 KiB
Go
package repo
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/google/uuid"
|
|
"github.com/hay-kot/content/backend/ent"
|
|
"github.com/hay-kot/content/backend/ent/documenttoken"
|
|
"github.com/hay-kot/content/backend/internal/types"
|
|
)
|
|
|
|
// DocumentTokensRepository is a repository for Document entity
|
|
type DocumentTokensRepository struct {
|
|
db *ent.Client
|
|
}
|
|
|
|
func (r *DocumentTokensRepository) Create(ctx context.Context, data types.DocumentTokenCreate) (*ent.DocumentToken, error) {
|
|
result, err := r.db.DocumentToken.Create().
|
|
SetDocumentID(data.DocumentID).
|
|
SetToken(data.TokenHash).
|
|
SetExpiresAt(data.ExpiresAt).
|
|
Save(ctx)
|
|
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return r.db.DocumentToken.Query().
|
|
Where(documenttoken.ID(result.ID)).
|
|
WithDocument().
|
|
Only(ctx)
|
|
}
|
|
|
|
func (r *DocumentTokensRepository) PurgeExpiredTokens(ctx context.Context) (int, error) {
|
|
return r.db.DocumentToken.Delete().Where(documenttoken.ExpiresAtLT(time.Now())).Exec(ctx)
|
|
}
|
|
|
|
func (r *DocumentTokensRepository) Delete(ctx context.Context, id uuid.UUID) error {
|
|
return r.db.DocumentToken.DeleteOneID(id).Exec(ctx)
|
|
}
|