forked from mirrors/homebox
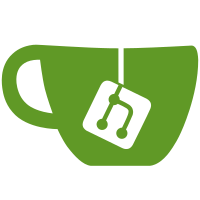
* change /content/ -> /homebox/ * add cache to code generators * update env variables to set data storage * update env variables * set env variables in prod container * implement attachment post route (WIP) * get attachment endpoint * attachment download * implement string utilities lib * implement generic drop zone * use explicit truncate * remove clean dir * drop strings composable for lib * update item types and add attachments * add attachment API * implement service context * consolidate API code * implement editing attachments * implement upload limit configuration * improve error handling * add docs for max upload size * fix test cases
76 lines
1.6 KiB
Go
76 lines
1.6 KiB
Go
package server
|
|
|
|
import (
|
|
"net/http"
|
|
)
|
|
|
|
type ValidationError struct {
|
|
Field string `json:"field"`
|
|
Reason string `json:"reason"`
|
|
}
|
|
|
|
type ValidationErrors []ValidationError
|
|
|
|
func (ve *ValidationErrors) HasErrors() bool {
|
|
if (ve == nil) || (len(*ve) == 0) {
|
|
return false
|
|
}
|
|
|
|
for _, err := range *ve {
|
|
if err.Field != "" {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (ve ValidationErrors) Append(field, reasons string) ValidationErrors {
|
|
return append(ve, ValidationError{
|
|
Field: field,
|
|
Reason: reasons,
|
|
})
|
|
}
|
|
|
|
// ErrorBuilder is a helper type to build a response that contains an array of errors.
|
|
// Typical use cases are for returning an array of validation errors back to the user.
|
|
//
|
|
// Example:
|
|
//
|
|
// {
|
|
// "errors": [
|
|
// "invalid id",
|
|
// "invalid name",
|
|
// "invalid description"
|
|
// ],
|
|
// "message": "Unprocessable Entity",
|
|
// "status": 422
|
|
// }
|
|
type ErrorBuilder struct {
|
|
errs []string
|
|
}
|
|
|
|
// HasErrors returns true if the ErrorBuilder has any errors.
|
|
func (eb *ErrorBuilder) HasErrors() bool {
|
|
if (eb.errs == nil) || (len(eb.errs) == 0) {
|
|
return false
|
|
}
|
|
return true
|
|
}
|
|
|
|
// AddError adds an error to the ErrorBuilder if an error is not nil. If the
|
|
// Error is nil, then nothing is added.
|
|
func (eb *ErrorBuilder) AddError(err error) {
|
|
if err != nil {
|
|
if eb.errs == nil {
|
|
eb.errs = make([]string, 0)
|
|
}
|
|
|
|
eb.errs = append(eb.errs, err.Error())
|
|
}
|
|
}
|
|
|
|
// Respond sends a JSON response with the ErrorBuilder's errors. If there are no errors, then
|
|
// the errors field will be an empty array.
|
|
func (eb *ErrorBuilder) Respond(w http.ResponseWriter, statusCode int) {
|
|
Respond(w, statusCode, Wrap(nil).AddError(http.StatusText(statusCode), eb.errs))
|
|
}
|