forked from mirrors/homebox
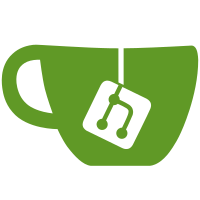
* remove empty services * remove old factory * remove old static files * cleanup more duplicate service code * file/folder reorg
42 lines
1.3 KiB
Go
42 lines
1.3 KiB
Go
package main
|
|
|
|
import (
|
|
"context"
|
|
"log"
|
|
"os"
|
|
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/migrate"
|
|
|
|
atlas "ariga.io/atlas/sql/migrate"
|
|
_ "ariga.io/atlas/sql/sqlite"
|
|
"entgo.io/ent/dialect"
|
|
"entgo.io/ent/dialect/sql/schema"
|
|
_ "github.com/mattn/go-sqlite3"
|
|
)
|
|
|
|
func main() {
|
|
ctx := context.Background()
|
|
// Create a local migration directory able to understand Atlas migration file format for replay.
|
|
dir, err := atlas.NewLocalDir("internal/data/migrations/migrations")
|
|
if err != nil {
|
|
log.Fatalf("failed creating atlas migration directory: %v", err)
|
|
}
|
|
// Migrate diff options.
|
|
opts := []schema.MigrateOption{
|
|
schema.WithDir(dir), // provide migration directory
|
|
schema.WithMigrationMode(schema.ModeReplay), // provide migration mode
|
|
schema.WithDialect(dialect.SQLite), // Ent dialect to use
|
|
schema.WithFormatter(atlas.DefaultFormatter),
|
|
schema.WithDropIndex(true),
|
|
schema.WithDropColumn(true),
|
|
}
|
|
if len(os.Args) != 2 {
|
|
log.Fatalln("migration name is required. Use: 'go run -mod=mod ent/migrate/main.go <name>'")
|
|
}
|
|
|
|
// Generate migrations using Atlas support for MySQL (note the Ent dialect option passed above).
|
|
err = migrate.NamedDiff(ctx, "sqlite://.data/homebox.migration.db?_fk=1", os.Args[1], opts...)
|
|
if err != nil {
|
|
log.Fatalf("failed generating migration file: %v", err)
|
|
}
|
|
}
|