forked from mirrors/homebox
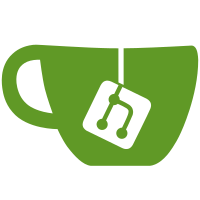
* change /content/ -> /homebox/ * add cache to code generators * update env variables to set data storage * update env variables * set env variables in prod container * implement attachment post route (WIP) * get attachment endpoint * attachment download * implement string utilities lib * implement generic drop zone * use explicit truncate * remove clean dir * drop strings composable for lib * update item types and add attachments * add attachment API * implement service context * consolidate API code * implement editing attachments * implement upload limit configuration * improve error handling * add docs for max upload size * fix test cases
42 lines
822 B
Go
42 lines
822 B
Go
package schema
|
|
|
|
import (
|
|
"entgo.io/ent"
|
|
"entgo.io/ent/schema/edge"
|
|
"entgo.io/ent/schema/field"
|
|
"github.com/hay-kot/homebox/backend/ent/schema/mixins"
|
|
)
|
|
|
|
// Attachment holds the schema definition for the Attachment entity.
|
|
type Attachment struct {
|
|
ent.Schema
|
|
}
|
|
|
|
func (Attachment) Mixin() []ent.Mixin {
|
|
return []ent.Mixin{
|
|
mixins.BaseMixin{},
|
|
}
|
|
}
|
|
|
|
// Fields of the Attachment.
|
|
func (Attachment) Fields() []ent.Field {
|
|
return []ent.Field{
|
|
field.Enum("type").
|
|
Values("photo", "manual", "warranty", "attachment").
|
|
Default("attachment"),
|
|
}
|
|
}
|
|
|
|
// Edges of the Attachment.
|
|
func (Attachment) Edges() []ent.Edge {
|
|
return []ent.Edge{
|
|
edge.From("item", Item.Type).
|
|
Ref("attachments").
|
|
Required().
|
|
Unique(),
|
|
edge.From("document", Document.Type).
|
|
Ref("attachments").
|
|
Required().
|
|
Unique(),
|
|
}
|
|
}
|