forked from mirrors/homebox
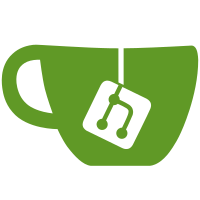
* implement custom http handler interface * implement trace_id * normalize http method spacing for consistent logs * fix failing test * fix linter errors * cleanup old dead code * more route cleanup * cleanup some inconsistent errors * update and generate code * make taskfile more consistent * update task calls * run tidy * drop `@` tag for version * use relative paths * tidy * fix auto-setting variables * update build paths * add contributing guide * tidy
39 lines
913 B
Go
39 lines
913 B
Go
package server
|
|
|
|
import (
|
|
"encoding/json"
|
|
"net/http"
|
|
)
|
|
|
|
type ErrorResponse struct {
|
|
Error string `json:"error"`
|
|
Fields map[string]string `json:"fields,omitempty"`
|
|
}
|
|
|
|
// Respond converts a Go value to JSON and sends it to the client.
|
|
// Adapted from https://github.com/ardanlabs/service/tree/master/foundation/web
|
|
func Respond(w http.ResponseWriter, statusCode int, data interface{}) error {
|
|
if statusCode == http.StatusNoContent {
|
|
w.WriteHeader(statusCode)
|
|
return nil
|
|
}
|
|
|
|
// Convert the response value to JSON.
|
|
jsonData, err := json.Marshal(data)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
// Set the content type and headers once we know marshaling has succeeded.
|
|
w.Header().Set("Content-Type", ContentJSON)
|
|
|
|
// Write the status code to the response.
|
|
w.WriteHeader(statusCode)
|
|
|
|
// Send the result back to the client.
|
|
if _, err := w.Write(jsonData); err != nil {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|