forked from mirrors/homebox
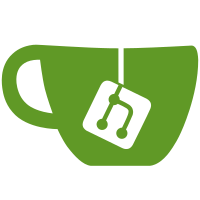
* rough implementation of WS based event system for server side notifications of mutation * fix test construction * fix deadlock on event bus * disable linter error * add item mutation events * remove old event bus code * refactor event system to use composables * refresh items table when new item is added * fix create form errors * cleanup unnecessary calls * fix importer erorrs + limit fn calls on import
44 lines
1 KiB
Go
44 lines
1 KiB
Go
package mid
|
|
|
|
import (
|
|
"bufio"
|
|
"errors"
|
|
"net"
|
|
"net/http"
|
|
|
|
"github.com/go-chi/chi/v5/middleware"
|
|
"github.com/rs/zerolog"
|
|
)
|
|
|
|
type spy struct {
|
|
http.ResponseWriter
|
|
status int
|
|
}
|
|
|
|
func (s *spy) WriteHeader(status int) {
|
|
s.status = status
|
|
s.ResponseWriter.WriteHeader(status)
|
|
}
|
|
|
|
func (s *spy) Hijack() (net.Conn, *bufio.ReadWriter, error) {
|
|
hj, ok := s.ResponseWriter.(http.Hijacker)
|
|
if !ok {
|
|
return nil, nil, errors.New("response writer does not support hijacking")
|
|
}
|
|
return hj.Hijack()
|
|
}
|
|
|
|
func Logger(l zerolog.Logger) func(http.Handler) http.Handler {
|
|
return func(h http.Handler) http.Handler {
|
|
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
|
|
reqID := r.Context().Value(middleware.RequestIDKey).(string)
|
|
|
|
l.Info().Str("method", r.Method).Str("path", r.URL.Path).Str("rid", reqID).Msg("request received")
|
|
|
|
s := &spy{ResponseWriter: w}
|
|
h.ServeHTTP(s, r)
|
|
|
|
l.Info().Str("method", r.Method).Str("path", r.URL.Path).Int("status", s.status).Str("rid", reqID).Msg("request finished")
|
|
})
|
|
}
|
|
}
|