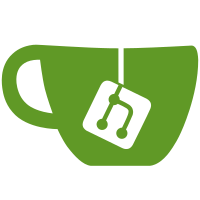
This change implements support for registry integration tests using the new py.test-based live server test fixture. We can now parametrize the protocols we use (in prep for V2_2), and it makes the code *much* cleaner and less hacky. Note that moving the vast majority of the tests over from the existing impl will come as a followup PR
35 lines
1.5 KiB
Python
35 lines
1.5 KiB
Python
from test.fixtures import *
|
|
from test.registry.liveserverfixture import *
|
|
from test.registry.fixtures import *
|
|
from test.registry.protocol_fixtures import *
|
|
|
|
from test.registry.protocols import Failures
|
|
|
|
|
|
def test_basic_push_pull(pusher, puller, basic_images, liveserver_session, app_reloader):
|
|
""" Test: Basic push and pull of an image to a new repository. """
|
|
credentials = ('devtable', 'password')
|
|
|
|
# Push a new repository.
|
|
pusher.push(liveserver_session, 'devtable', 'newrepo', 'latest', basic_images,
|
|
credentials=credentials)
|
|
|
|
# Pull the repository to verify.
|
|
puller.pull(liveserver_session, 'devtable', 'newrepo', 'latest', basic_images,
|
|
credentials=credentials)
|
|
|
|
|
|
def test_push_invalid_credentials(pusher, basic_images, liveserver_session, app_reloader):
|
|
""" Test: Ensure we get auth errors when trying to push with invalid credentials. """
|
|
invalid_credentials = ('devtable', 'notcorrectpassword')
|
|
|
|
pusher.push(liveserver_session, 'devtable', 'newrepo', 'latest', basic_images,
|
|
credentials=invalid_credentials, expected_failure=Failures.UNAUTHENTICATED)
|
|
|
|
|
|
def test_pull_invalid_credentials(puller, basic_images, liveserver_session, app_reloader):
|
|
""" Test: Ensure we get auth errors when trying to pull with invalid credentials. """
|
|
invalid_credentials = ('devtable', 'notcorrectpassword')
|
|
|
|
puller.pull(liveserver_session, 'devtable', 'newrepo', 'latest', basic_images,
|
|
credentials=invalid_credentials, expected_failure=Failures.UNAUTHENTICATED)
|